
Summary: This tutorial demonstrates several ways of interpolating with FSharp.Stats
With the FSharp.Stats.Interpolation
module you can apply various interpolation methods. While interpolating functions always go through the input points (knots), methods to predict function values
from x values (or x vectors in multivariate interpolation) not contained in the input, vary greatly. A Interpolation
type provides many common methods for interpolation of two dimensional data. These include
- Linear spline interpolation (connecting knots by straight lines)
- Polynomial interpolation
- Hermite spline interpolation
- Cubic spline interpolation with 5 boundary conditions
- Akima subspline interpolation
The following code snippet summarizes all interpolation methods. In the following sections, every method is discussed in detail!
open Plotly.NET
open FSharp.Stats
let testDataX = [|1. .. 10.|]
let testDataY = [|0.5;-1.;0.;0.;0.;0.;1.;1.;3.;3.5|]
let coefStep = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.Step) // step function
let coefLinear = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.LinearSpline) // Straight lines passing all points
let coefAkima = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.AkimaSubSpline) // Akima cubic subspline
let coefCubicNa = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.CubicSpline Interpolation.CubicSpline.BoundaryCondition.Natural) // cubic spline with f'' at borders is set to 0
let coefCubicPe = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.CubicSpline Interpolation.CubicSpline.BoundaryCondition.Periodic) // cubic spline with equal f' at borders
let coefCubicNo = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.CubicSpline Interpolation.CubicSpline.BoundaryCondition.NotAKnot) // cubic spline with continous f''' at second and penultimate knot
let coefCubicPa = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.CubicSpline Interpolation.CubicSpline.BoundaryCondition.Parabolic) // cubic spline with quadratic polynomial at borders
let coefCubicCl = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.CubicSpline (Interpolation.CubicSpline.BoundaryCondition.Clamped (0,-1))) // cubic spline with border f' set to 0 and -1
let coefHermite = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.HermiteSpline HermiteMethod.CSpline)
let coefHermiteMono = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.HermiteSpline HermiteMethod.PreserveMonotonicity)
let coefHermiteSlop = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.HermiteSpline (HermiteMethod.WithSlopes (vector [0.;0.;0.;0.;0.;0.;0.;0.;0.;0.])))
let coefPolynomial = Interpolation.interpolate(testDataX,testDataY,InterpolationMethod.Polynomial) // interpolating polynomial
let coefApproximate = Interpolation.Approximation.approxWithPolynomialFromValues(testDataX,testDataY,10,Interpolation.Approximation.Spacing.Chebyshev) //interpolating polynomial of degree 9 with knots spaced according to Chebysehv
let interpolationComparison =
[
Chart.Point(testDataX,testDataY,Name="data")
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefStep) x) |> Chart.Line |> Chart.withTraceInfo "Step"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefLinear) x) |> Chart.Line |> Chart.withTraceInfo "Linear"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefAkima) x) |> Chart.Line |> Chart.withTraceInfo "Akima"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefCubicNa) x) |> Chart.Line |> Chart.withTraceInfo "Cubic_natural"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefCubicPe) x) |> Chart.Line |> Chart.withTraceInfo "Cubic_periodic"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefCubicNo) x) |> Chart.Line |> Chart.withTraceInfo "Cubic_notaknot"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefCubicPa) x) |> Chart.Line |> Chart.withTraceInfo "Cubic_parabolic"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefCubicCl) x) |> Chart.Line |> Chart.withTraceInfo "Cubic_clamped"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefHermite) x) |> Chart.Line |> Chart.withTraceInfo "Hermite cSpline"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefHermiteMono) x) |> Chart.Line |> Chart.withTraceInfo "Hermite monotone"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefHermiteSlop) x) |> Chart.Line |> Chart.withTraceInfo "Hermite slope"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,Interpolation.predict(coefPolynomial) x) |> Chart.Line |> Chart.withTraceInfo "Polynomial"
[1. .. 0.01 .. 10.] |> List.map (fun x -> x,coefApproximate.Predict x) |> Chart.Line |> Chart.withTraceInfo "Chebyshev"
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle("x data")
|> Chart.withYAxisStyle("y data")
|> Chart.withSize(800.,600.)
Here a polynomial is fitted to the data. In general, a polynomial with degree = dataPointNumber - 1 has sufficient flexibility to interpolate all data points.
The least squares approach is not sufficient to converge to an interpolating polynomial! A degree other than n-1 results in a regression polynomial.
open Plotly.NET
open FSharp.Stats
let xData = vector [|1.;2.;3.;4.;5.;6.|]
let yData = vector [|4.;7.;9.;8.;7.;9.;|]
//Polynomial interpolation
//Define the polynomial coefficients. In Interpolation the order is equal to the data length - 1.
let coefficients =
Interpolation.Polynomial.interpolate xData yData
let interpolFunction x =
Interpolation.Polynomial.predict coefficients x
let rawChart =
Chart.Point(xData,yData)
|> Chart.withTraceInfo "raw data"
let interpolPol =
let fit = [|1. .. 0.1 .. 6.|] |> Array.map (fun x -> x,interpolFunction x)
fit
|> Chart.Line
|> Chart.withTraceInfo "interpolating polynomial"
let chartPol =
[rawChart;interpolPol]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
Splines are flexible strips of wood, that were used by shipbuilders to draw smooth shapes. In graphics and mathematics a piecewise cubic polynomial (order = 3) is called spline.
The curvature (second derivative) of a cubic polynomial is proportional to its tense energy and in spline theory the curvature is minimized. Therefore, the resulting function is very smooth.
To solve for the spline coefficients it is necessary to define two additional constraints, so called boundary conditions:
natural spline (most used spline variant): f''
at borders is set to 0
periodic spline: f'
at first point is the same as f'
at the last point
parabolic spline: f''
at first/second and last/penultimate knot are equal
notAKnot spline: f'''
at second and penultimate knot are continuous
- quadratic spline: first and last polynomial are quadratic, not cubic
clamped spline: f'
at first and last knot are set by user
In general, piecewise cubic splines only are defined within the region defined by the used x values. Using predict
with x values outside this range, uses the slopes and intersects of the nearest knot and utilizes them for prediction.
open Plotly.NET
open FSharp.Stats.Interpolation
let xValues = vector [1.;2.;3.;4.;5.5;6.]
let yValues = vector [1.;8.;6.;3.;7.;1.]
//calculates the spline coefficients for a natural spline
let coeffSpline =
CubicSpline.interpolate CubicSpline.BoundaryCondition.Natural xValues yValues
//cubic interpolating splines are only defined within the region defined in xValues
let interpolateFunctionWithinRange x =
CubicSpline.predictWithinRange coeffSpline x
//to interpolate x_Values that are out of the region defined in xValues
//interpolates the interpolation spline with linear prediction at borderknots
let interpolateFunction x =
CubicSpline.predict coeffSpline x
//to compare the spline interpolate with an interpolating polynomial:
let coeffPolynomial =
Interpolation.Polynomial.interpolate xValues yValues
let interpolateFunctionPol x =
Interpolation.Polynomial.predict coeffPolynomial x
//A linear spline draws straight lines to interpolate all data
let coeffLinearSpline = Interpolation.LinearSpline.interpolate (Array.ofSeq xValues) (Array.ofSeq yValues)
let interpolateFunctionLinSp = Interpolation.LinearSpline.predict coeffLinearSpline
let splineChart =
[
Chart.Point(xValues,yValues) |> Chart.withTraceInfo "raw data"
[ 1. .. 0.1 .. 6.] |> List.map (fun x -> x,interpolateFunctionPol x) |> Chart.Line |> Chart.withTraceInfo "fitPolynomial"
[-1. .. 0.1 .. 8.] |> List.map (fun x -> x,interpolateFunction x) |> Chart.Line |> Chart.withLineStyle(Dash=StyleParam.DrawingStyle.Dash) |> Chart.withTraceInfo "fitSpline"
[ 1. .. 0.1 .. 6.] |> List.map (fun x -> x,interpolateFunctionWithinRange x)|> Chart.Line |> Chart.withTraceInfo "fitSpline_withinRange"
[ 1. .. 0.1 .. 6.] |> List.map (fun x -> x,interpolateFunctionLinSp x) |> Chart.Line |> Chart.withTraceInfo "fitLinearSpline"
]
|> Chart.combine
|> Chart.withTitle "Interpolation methods"
|> Chart.withTemplate ChartTemplates.lightMirrored
//additionally you can calculate the derivatives of the spline
//The cubic spline interpolation is continuous in f, f', and f''.
let derivativeChart =
[
Chart.Point(xValues,yValues) |> Chart.withTraceInfo "raw data"
[1. .. 0.1 .. 6.] |> List.map (fun x -> x,interpolateFunction x) |> Chart.Line |> Chart.withTraceInfo "spline fit"
[1. .. 0.1 .. 6.] |> List.map (fun x -> x,CubicSpline.getFirstDerivative coeffSpline x) |> Chart.Point |> Chart.withTraceInfo "fst derivative"
[1. .. 0.1 .. 6.] |> List.map (fun x -> x,CubicSpline.getSecondDerivative coeffSpline x) |> Chart.Point |> Chart.withTraceInfo "snd derivative"
[1. .. 0.1 .. 6.] |> List.map (fun x -> x,CubicSpline.getThirdDerivative coeffSpline x) |> Chart.Point |> Chart.withTraceInfo "trd derivative"
]
|> Chart.combine
|> Chart.withTitle "Cubic spline derivatives"
|> Chart.withTemplate ChartTemplates.lightMirrored
Akima subsplines are highly connected to default cubic spline interpolation. The main difference is the missing constraint of curvature continuity. This enhanced curvature flexibility diminishes oscillations of the
interpolating piecewise cubic subsplines. Subsplines differ from regular splines because they are discontinuous in the second derivative. See http://www.dorn.org/uni/sls/kap06/f08_0204.htm for more information.
let xVal = [|1. .. 10.|]
let yVal = [|1.;-0.5;2.;2.;2.;3.;3.;3.;5.;4.|]
let akimaCoeffs = Akima.interpolate xVal yVal
let akima =
[0. .. 0.1 .. 11.]
|> List.map (fun x ->
x,Akima.predict akimaCoeffs x)
|> Chart.Line
let cubicCoeffs = CubicSpline.interpolate CubicSpline.BoundaryCondition.Natural (vector xVal) (vector yVal)
let cubicSpline =
[0. .. 0.1 .. 11.]
|> List.map (fun x ->
x,CubicSpline.predict cubicCoeffs x)
|> Chart.Line
let akimaChart =
[
Chart.Point(xVal,yVal,Name="data")
cubicSpline |> Chart.withTraceInfo "cubic spline"
akima |> Chart.withTraceInfo "akima spline"
]
|> Chart.combine
|> Chart.withTitle "Cubic spline derivatives"
|> Chart.withTemplate ChartTemplates.lightMirrored
In Hermite interpolation the user can define the slopes of the function in the knots. This is especially useful if the function is oscillating and thereby generates local minima/maxima.
Intuitively the slope of a knot should be between the slopes of the adjacent straight lines. By using this slope calculation a monotone knot behavior results in a monotone spline.
open FSharp.Stats
open FSharp.Stats.Interpolation
open Plotly.NET
//example from http://www.korf.co.uk/spline.pdf
let xDataH = vector [0.;10.;30.;50.;70.;80.;82.]
let yDataH = vector [150.;200.;200.;200.;180.;100.;0.]
//Get slopes for Hermite spline. Try to interpolate a monotone function.
let tryMonotoneSlope = CubicSpline.Hermite.interpolatePreserveMonotonicity xDataH yDataH
//get function for Hermite spline
let funHermite = fun x -> CubicSpline.Hermite.predict tryMonotoneSlope x
//get coefficients and function for a classic natural spline
let coeffSpl = CubicSpline.interpolate CubicSpline.BoundaryCondition.Natural xDataH yDataH
let funNaturalSpline x = CubicSpline.predict coeffSpl x
//get coefficients and function for a classic polynomial interpolation
let coeffPolInterpol =
//let neutralWeights = Vector.init 7 (fun x -> 1.)
//Fitting.LinearRegression.OLS.Polynomial.coefficientsWithWeighting 6 neutralWeights xDataH yDataH
Interpolation.Polynomial.interpolate xDataH yDataH
let funPolInterpol x =
//Fitting.LinearRegression.OLS.Polynomial.fit 6 coeffPolInterpol x
Interpolation.Polynomial.predict coeffPolInterpol x
let splineComparison =
[
Chart.Point(xDataH,yDataH) |> Chart.withTraceInfo "raw data"
[0. .. 82.] |> List.map (fun x -> x,funNaturalSpline x) |> Chart.Line |> Chart.withTraceInfo "natural spline"
[0. .. 82.] |> List.map (fun x -> x,funHermite x ) |> Chart.Line |> Chart.withTraceInfo "hermite spline"
[0. .. 82.] |> List.map (fun x -> x,funPolInterpol x ) |> Chart.Line |> Chart.withTraceInfo "polynomial"
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
In Bezier interpolation the user can define control points in order to interpolate between points. The first and last point (within the given coordinate sequence) are interpolated, while all others serve as control points that stretch the connection.
If there is just one control point (coordinate collection length=3) the resulting curve is quadratic, two control points create a cubic curve etc.. Nested LERPs are used to identify the desired y-value from an given x value.
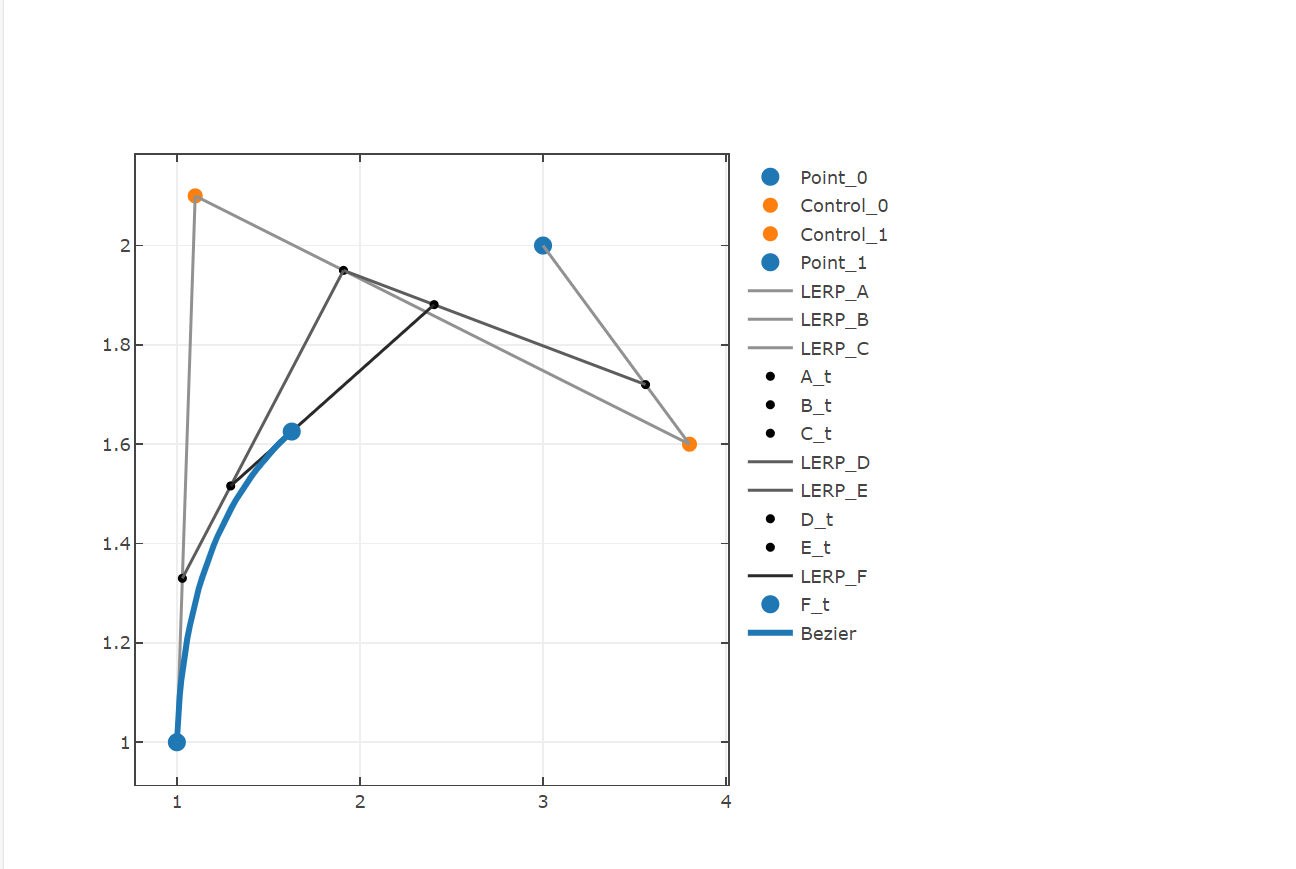
open FSharp.Stats
open FSharp.Stats.Interpolation
open Plotly.NET
let bezierInterpolation =
let t = 0.3
let p0 = vector [|3.;0.|] //point 0 that should be traversed
let c0 = vector [|-1.5;8.|] //control point 0
let c1 = vector [|1.5;9.|] //control point 1
let c2 = vector [|6.5;-1.5|] //control point 2
let c3 = vector [|13.5;4.|] //control point 3
let p1 = vector [|10.;5.|] //point 1 that should be traversed
let toPoint (v : vector) = v[0],v[1]
let interpolate = Bezier.interpolate [|p0;c0;c1;c2;c3;p1|] >> toPoint
[
Chart.Point([p0.[0]],[p0.[1]],Name="Point_0",MarkerColor=Color.fromHex "#1f77b4") |> Chart.withMarkerStyle(Size=12)
Chart.Point([c0.[0]],[c0.[1]],Name="Control_0",MarkerColor=Color.fromHex "#ff7f0e")|> Chart.withMarkerStyle(Size=10)
Chart.Point([c1.[0]],[c1.[1]],Name="Control_1",MarkerColor=Color.fromHex "#ff7f0e")|> Chart.withMarkerStyle(Size=10)
Chart.Point([c2.[0]],[c2.[1]],Name="Control_2",MarkerColor=Color.fromHex "#ff7f0e")|> Chart.withMarkerStyle(Size=10)
Chart.Point([c3.[0]],[c3.[1]],Name="Control_3",MarkerColor=Color.fromHex "#ff7f0e")|> Chart.withMarkerStyle(Size=10)
Chart.Point([p1.[0]],[p1.[1]],Name="Point_1",MarkerColor=Color.fromHex "#1f77b4") |> Chart.withMarkerStyle(Size=12)
[0. .. 0.01 .. 1.] |> List.map interpolate |> Chart.Line |> Chart.withTraceInfo "Bezier" |> Chart.withLineStyle(Color=Color.fromHex "#1f77b4")
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
Bezier interpolation is not limited to 2D points, it can be also be used to interpolate vectors.
let bezierInterpolation3d =
let p0 = vector [|1.;1.;1.|] //point 0 that should be traversed
let c0 = vector [|1.5;2.1;2.|] //control point 0
let c1 = vector [|5.8;1.6;1.4|] //control point 1
let p1 = vector [|3.;2.;0.|] //point 1 that should be traversed
let to3Dpoint (v : vector) = v[0],v[1],v[2]
let interpolate = Bezier.interpolate [|p0;c0;c1;p1|] >> to3Dpoint
[
Chart.Point3D([p0.[0]],[p0.[1]],[p0.[2]],Name="Point_0",MarkerColor=Color.fromHex "#1f77b4") |> Chart.withMarkerStyle(Size=12)
Chart.Point3D([c0.[0]],[c0.[1]],[c0.[2]],Name="Control_0",MarkerColor=Color.fromHex "#ff7f0e")|> Chart.withMarkerStyle(Size=10)
Chart.Point3D([c1.[0]],[c1.[1]],[c1.[2]],Name="Control_1",MarkerColor=Color.fromHex "#ff7f0e")|> Chart.withMarkerStyle(Size=10)
Chart.Point3D([p1.[0]],[p1.[1]],[p1.[2]],Name="Point_1",MarkerColor=Color.fromHex "#1f77b4") |> Chart.withMarkerStyle(Size=12)
[0. .. 0.01 .. 1.] |> List.map interpolate |> Chart.Line3D |> Chart.withTraceInfo "Bezier" |> Chart.withLineStyle(Color=Color.fromHex "#1f77b4",Width=10.)
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
Polynomials are great when it comes to slope/area determination or the investigation of signal properties.
When faced with an unknown (or complex) function it may be beneficial to approximate the data using polynomials, even if it does not correspond to the real model.
Polynomial regression can cause difficulties if the signal is flexible and the required polynomial degree is high. Floating point errors sometimes lead to vanishing coefficients and even though the
SSE should decrease, it does not and a strange, squiggly shape is generated.
Polynomial interpolation can help to obtain a robust polynomial description of the data, but is prone to Runges phenomenon.
In the next section, data is introduced that should be converted to a polynomial approximation.
let xs = [|0. .. 0.2 .. 3.|]
let ys = [|5.;5.5;6.;6.1;4.;1.;0.7;0.3;0.5;0.9;5.;9.;9.;8.;6.5;5.;|]
let chebyChart =
Chart.Line(xs,ys,Name="raw",ShowMarkers=true)
|> Chart.withTemplate ChartTemplates.lightMirrored
Let's fit a interpolating polynomial to the points:
// calculates the coefficients of the interpolating polynomial
let coeffs =
Interpolation.Polynomial.interpolate (vector xs) (vector ys)
// determines the y value of a given x value with the interpolating coefficients
let interpolatingFunction x =
Interpolation.Polynomial.predict coeffs x
// plot the interpolated data
let interpolChart =
let ys_interpol =
[|0. .. 0.01 .. 3.|]
|> Seq.map (fun x -> x,interpolatingFunction x)
Chart.Line(ys_interpol,Name="interpol")
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "xs"
|> Chart.withYAxisStyle "ys"
let cbChart =
[
chebyChart
interpolChart
]
|> Chart.combine
Because of Runges phenomenon the interpolating polynomial overshoots in the outer areas of the data. It would be detrimental if this function approximation is used to investigate signal properties.
To reduce this overfitting you can use x axis nodes that are spaced according to Chebyshev. Here, nodes are sparse in the center of the analysed function and are more dense in the outer areas.
// new x values are determined in the x axis range of the data. These should reduce overshooting behaviour.
// since the original data consisted of 16 points, 16 nodes are initialized
let xs_cheby =
Interpolation.Approximation.chebyshevNodes (Interval.CreateClosed<float>(0.,3.)) 16
// to get the corresponding y values to the xs_cheby a linear spline is generated that approximates the new y values
let ys_cheby =
let ls = Interpolation.LinearSpline.interpolate xs ys
xs_cheby |> Vector.map (Interpolation.LinearSpline.predict ls)
// again polynomial interpolation coefficients are determined, but here with the x and y data that correspond to the chebyshev spacing
let coeffs_cheby = Interpolation.Polynomial.interpolate xs_cheby ys_cheby
// Note: the upper panel can be summarized by the follwing function:
Interpolation.Approximation.approxWithPolynomialFromValues(xData=xs,yData=ys,n=16,spacing=Approximation.Spacing.Chebyshev)
Using the determined polynomial coefficients, the standard approach for fitting can be used to plot the signal together with the function approximation. Obviously the example data
is difficult to approximate, but the chebyshev spacing of the x-nodes drastically reduces the overfitting in the outer areas of the signal.
// function using the cheby_coefficients to get y values of given x value
let interpolating_cheby x = Interpolation.Polynomial.predict coeffs_cheby x
let interpolChart_cheby =
let ys_interpol_cheby =
vector [|0. .. 0.01 .. 3.|]
|> Seq.map (fun x -> x,interpolating_cheby x)
Chart.Line(ys_interpol_cheby,Name="interpol_cheby")
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "xs"
|> Chart.withYAxisStyle "ys"
let cbChart_cheby =
[
chebyChart
interpolChart
Chart.Line(xs_cheby,ys_cheby,ShowMarkers=true,Name="cheby_nodes") |> Chart.withTemplate ChartTemplates.lightMirrored|> Chart.withXAxisStyle "xs"|> Chart.withYAxisStyle "ys"
interpolChart_cheby
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "xs"
|> Chart.withYAxisStyle "ys"
If a non-polynomal function should be approximated as polynomial you can use Interpolation.Approximation.approxWithPolynomial
with specifying the interval in which the function should be approximated.
namespace Plotly
namespace Plotly.NET
module Defaults
from Plotly.NET
<summary>
Contains mutable global default values.
Changing these values will apply the default values to all consecutive Chart generations.
</summary>
val mutable DefaultDisplayOptions: DisplayOptions
Multiple items
type DisplayOptions =
inherit DynamicObj
new: unit -> DisplayOptions
static member addAdditionalHeadTags: additionalHeadTags: XmlNode list -> (DisplayOptions -> DisplayOptions)
static member addDescription: description: XmlNode list -> (DisplayOptions -> DisplayOptions)
static member combine: first: DisplayOptions -> second: DisplayOptions -> DisplayOptions
static member getAdditionalHeadTags: displayOpts: DisplayOptions -> XmlNode list
static member getDescription: displayOpts: DisplayOptions -> XmlNode list
static member getPlotlyReference: displayOpts: DisplayOptions -> PlotlyJSReference
static member init: ?AdditionalHeadTags: XmlNode list * ?Description: XmlNode list * ?PlotlyJSReference: PlotlyJSReference -> DisplayOptions
static member initCDNOnly: unit -> DisplayOptions
...
--------------------
new: unit -> DisplayOptions
static member DisplayOptions.init: ?AdditionalHeadTags: Giraffe.ViewEngine.HtmlElements.XmlNode list * ?Description: Giraffe.ViewEngine.HtmlElements.XmlNode list * ?PlotlyJSReference: PlotlyJSReference -> DisplayOptions
type PlotlyJSReference =
| CDN of string
| Full
| Require of string
| NoReference
<summary>
Sets how plotly is referenced in the head of html docs.
</summary>
union case PlotlyJSReference.NoReference: PlotlyJSReference
Multiple items
namespace FSharp
--------------------
namespace Microsoft.FSharp
namespace FSharp.Stats
val testDataX: float[]
val testDataY: float[]
val coefStep: InterpolationCoefficients
Multiple items
module Interpolation
from FSharp.Stats
<summary>
This module contains functionalities to perform various interpolation methods for two dimensional data.
</summary>
--------------------
type Interpolation =
new: unit -> Interpolation
static member getFirstDerivative: coef: InterpolationCoefficients -> (float -> float)
static member getIntegralBetween: coef: InterpolationCoefficients * x1: float * x2: float -> float
static member getSecondDerivative: coef: InterpolationCoefficients -> (float -> float)
static member interpolate: xValues: float array * yValues: float array * method: InterpolationMethod -> InterpolationCoefficients
static member predict: coef: InterpolationCoefficients -> (float -> float)
<summary>
This type contains functionalities to perform various interpolation methods for two dimensional data. It summarizes functions contained within the interpolation module.
</summary>
--------------------
new: unit -> Interpolation
static member Interpolation.interpolate: xValues: float array * yValues: float array * method: InterpolationMethod -> InterpolationCoefficients
type InterpolationMethod =
| Step
| LinearSpline
| Polynomial
| CubicSpline of BoundaryCondition
| AkimaSubSpline
| HermiteSpline of HermiteMethod
<summary>
Lets the user choose between 5 interpolation methods. One simply connects dots (LinearSpline), one forms a single interpolating polynomial (Polynomial, and three of them employ piecewise cubic polynomials.
</summary>
union case InterpolationMethod.Step: InterpolationMethod
<summary>
Creates a step function from x,y coordinates. Coordinates are interpolated by straight horizontal lines between two knots.
</summary>
val coefLinear: InterpolationCoefficients
union case InterpolationMethod.LinearSpline: InterpolationMethod
<summary>
Creates a linear spline from x,y coordinates. x,y coordinates are interpolated by straight lines between two knots.
Equivalent to interval-wise simple linear regression between any neighbouring pair of data.
</summary>
val coefAkima: InterpolationCoefficients
union case InterpolationMethod.AkimaSubSpline: InterpolationMethod
<summary>
Creates a subspline as piecewise cubic polynomials with continuous first derivative but DIScontinuous second derivative at the knots.
</summary>
val coefCubicNa: InterpolationCoefficients
union case InterpolationMethod.CubicSpline: Interpolation.CubicSpline.BoundaryCondition -> InterpolationMethod
<summary>
Creates a spline as piecewise cubic polynomials with continuous first and second derivative at the knots.
</summary>
<param name="CubicSpline.BoundaryCondition">One of four conditions to manipulate the curvatures at the outer knots.</param>
module CubicSpline
from FSharp.Stats.InterpolationModule
<summary>
Cubic splines interpolate two dimensional data by applying piecewise cubic polynomials that are continuous at the input coordinates (knots).
The function itself, the first- and second derivative are continuous at the knots.
</summary>
type BoundaryCondition =
| Natural
| Periodic
| Parabolic
| NotAKnot
| Quadratic
| Clamped of (float * float)
| SecondDerivative of (float * float)
union case Interpolation.CubicSpline.BoundaryCondition.Natural: Interpolation.CubicSpline.BoundaryCondition
<summary>most used spline variant: f'' at borders is set to 0</summary>
val coefCubicPe: InterpolationCoefficients
union case Interpolation.CubicSpline.BoundaryCondition.Periodic: Interpolation.CubicSpline.BoundaryCondition
<summary>
f' at first point is the same as f' at the last point
</summary>
val coefCubicNo: InterpolationCoefficients
union case Interpolation.CubicSpline.BoundaryCondition.NotAKnot: Interpolation.CubicSpline.BoundaryCondition
<summary>
f''' at second and penultimate knot are continuous
</summary>
val coefCubicPa: InterpolationCoefficients
union case Interpolation.CubicSpline.BoundaryCondition.Parabolic: Interpolation.CubicSpline.BoundaryCondition
<summary>
f'' at first/second and last/penultimate knot are equal
</summary>
val coefCubicCl: InterpolationCoefficients
union case Interpolation.CubicSpline.BoundaryCondition.Clamped: (float * float) -> Interpolation.CubicSpline.BoundaryCondition
<summary>
f' at first and last knot are set by user
</summary>
val coefHermite: InterpolationCoefficients
union case InterpolationMethod.HermiteSpline: HermiteMethod -> InterpolationMethod
<summary>
Creates a spline as piecewise cubic polynomials with given slope.
</summary>
<param name="HermiteMethod">choose between cSpline, given slopes or monotonicity when appropriate</param>
type HermiteMethod =
| CSpline
| WithSlopes of vector
| PreserveMonotonicity
union case HermiteMethod.CSpline: HermiteMethod
<summary>
Default hermite spline (cSpline)
</summary>
val coefHermiteMono: InterpolationCoefficients
union case HermiteMethod.PreserveMonotonicity: HermiteMethod
<summary>
Creates a spline from x,y coordinates. x,y coordinates are interpolated by cubic polynomials between two knots.
If a region in the raw data is monotone, the resulting interpolator is monotone as well.
</summary>
val coefHermiteSlop: InterpolationCoefficients
union case HermiteMethod.WithSlopes: vector -> HermiteMethod
<summary>
Creates a spline from x,y coordinates. x,y coordinates are interpolated by cubic polynomialsbetween two knots.
The slope at each knot is defined by a input slope vector.
</summary>
Multiple items
val vector: l: seq<float> -> Vector<float>
--------------------
type vector = Vector<float>
val coefPolynomial: InterpolationCoefficients
union case InterpolationMethod.Polynomial: InterpolationMethod
<summary>
Creates a polynomial of degree n-1 that interpolate all n knots.
</summary>
val coefApproximate: Interpolation.Polynomial.PolynomialCoef
Multiple items
module Approximation
from FSharp.Stats.InterpolationModule
<summary>
Approximation
</summary>
--------------------
type Approximation =
new: unit -> Approximation
static member approxWithPolynomial: f: (float -> float) * i: Interval<float> * n: int * spacing: Spacing -> PolynomialCoef
static member approxWithPolynomialFromValues: xData: seq<float> * yData: seq<float> * n: int * spacing: Spacing -> PolynomialCoef
--------------------
new: unit -> Interpolation.Approximation
static member Interpolation.Approximation.approxWithPolynomialFromValues: xData: seq<float> * yData: seq<float> * n: int * spacing: Interpolation.Approximation.Spacing -> Interpolation.Polynomial.PolynomialCoef
type Spacing =
| Equally
| Chebyshev
union case Interpolation.Approximation.Spacing.Chebyshev: Interpolation.Approximation.Spacing
val interpolationComparison: GenericChart.GenericChart
type Chart =
static member AnnotatedHeatmap: zData: seq<#seq<'a1>> * annotationText: seq<#seq<string>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?X: seq<'a3> * ?MultiX: seq<seq<'a3>> * ?XGap: int * ?Y: seq<'a4> * ?MultiY: seq<seq<'a4>> * ?YGap: int * ?Text: 'a5 * ?MultiText: seq<'a5> * ?ColorBar: ColorBar * ?ColorScale: Colorscale * ?ShowScale: bool * ?ReverseScale: bool * ?ZSmooth: SmoothAlg * ?Transpose: bool * ?UseWebGL: bool * ?ReverseYAxis: bool * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a3 :> IConvertible and 'a4 :> IConvertible and 'a5 :> IConvertible) + 1 overload
static member Area: x: seq<#IConvertible> * y: seq<#IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: MarkerSymbol * ?MultiMarkerSymbol: seq<MarkerSymbol> * ?Marker: Marker * ?LineColor: Color * ?LineColorScale: Colorscale * ?LineWidth: float * ?LineDash: DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: Orientation * ?GroupNorm: GroupNorm * ?FillColor: Color * ?FillPatternShape: PatternShape * ?FillPattern: Pattern * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart (requires 'a2 :> IConvertible) + 1 overload
static member Bar: values: seq<#IConvertible> * ?Keys: seq<'a1> * ?MultiKeys: seq<seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerPatternShape: PatternShape * ?MultiMarkerPatternShape: seq<PatternShape> * ?MarkerPattern: Pattern * ?Marker: Marker * ?Base: #IConvertible * ?Width: 'a4 * ?MultiWidth: seq<'a4> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a4 :> IConvertible) + 1 overload
static member BoxPlot: ?X: seq<'a0> * ?MultiX: seq<seq<'a0>> * ?Y: seq<'a1> * ?MultiY: seq<seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Text: 'a2 * ?MultiText: seq<'a2> * ?FillColor: Color * ?MarkerColor: Color * ?Marker: Marker * ?Opacity: float * ?WhiskerWidth: float * ?BoxPoints: BoxPoints * ?BoxMean: BoxMean * ?Jitter: float * ?PointPos: float * ?Orientation: Orientation * ?OutlineColor: Color * ?OutlineWidth: float * ?Outline: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?Notched: bool * ?NotchWidth: float * ?QuartileMethod: QuartileMethod * ?UseDefaults: bool -> GenericChart (requires 'a0 :> IConvertible and 'a1 :> IConvertible and 'a2 :> IConvertible) + 2 overloads
static member Bubble: x: seq<#IConvertible> * y: seq<#IConvertible> * sizes: seq<int> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: MarkerSymbol * ?MultiMarkerSymbol: seq<MarkerSymbol> * ?Marker: Marker * ?LineColor: Color * ?LineColorScale: Colorscale * ?LineWidth: float * ?LineDash: DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: Orientation * ?GroupNorm: GroupNorm * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart (requires 'a2 :> IConvertible) + 1 overload
static member Candlestick: ``open`` : seq<#IConvertible> * high: seq<#IConvertible> * low: seq<#IConvertible> * close: seq<#IConvertible> * ?X: seq<'a4> * ?MultiX: seq<seq<'a4>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?Text: 'a5 * ?MultiText: seq<'a5> * ?Line: Line * ?IncreasingColor: Color * ?Increasing: FinanceMarker * ?DecreasingColor: Color * ?Decreasing: FinanceMarker * ?WhiskerWidth: float * ?ShowXAxisRangeSlider: bool * ?UseDefaults: bool -> GenericChart (requires 'a4 :> IConvertible and 'a5 :> IConvertible) + 2 overloads
static member Column: values: seq<#IConvertible> * ?Keys: seq<'a1> * ?MultiKeys: seq<seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerPatternShape: PatternShape * ?MultiMarkerPatternShape: seq<PatternShape> * ?MarkerPattern: Pattern * ?Marker: Marker * ?Base: #IConvertible * ?Width: 'a4 * ?MultiWidth: seq<'a4> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a4 :> IConvertible) + 1 overload
static member Contour: zData: seq<#seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?X: seq<'a2> * ?MultiX: seq<seq<'a2>> * ?Y: seq<'a3> * ?MultiY: seq<seq<'a3>> * ?Text: 'a4 * ?MultiText: seq<'a4> * ?ColorBar: ColorBar * ?ColorScale: Colorscale * ?ShowScale: bool * ?ReverseScale: bool * ?Transpose: bool * ?ContourLineColor: Color * ?ContourLineDash: DrawingStyle * ?ContourLineSmoothing: float * ?ContourLine: Line * ?ContoursColoring: ContourColoring * ?ContoursOperation: ConstraintOperation * ?ContoursType: ContourType * ?ShowContourLabels: bool * ?ContourLabelFont: Font * ?Contours: Contours * ?FillColor: Color * ?NContours: int * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a3 :> IConvertible and 'a4 :> IConvertible)
static member Funnel: x: seq<#IConvertible> * y: seq<#IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?Width: float * ?Offset: float * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?Orientation: Orientation * ?AlignmentGroup: string * ?OffsetGroup: string * ?MarkerColor: Color * ?MarkerOutline: Line * ?Marker: Marker * ?TextInfo: TextInfo * ?ConnectorLineColor: Color * ?ConnectorLineStyle: DrawingStyle * ?ConnectorFillColor: Color * ?ConnectorLine: Line * ?Connector: FunnelConnector * ?InsideTextFont: Font * ?OutsideTextFont: Font * ?UseDefaults: bool -> GenericChart (requires 'a2 :> IConvertible)
static member Heatmap: zData: seq<#seq<'a1>> * ?X: seq<'a2> * ?MultiX: seq<seq<'a2>> * ?Y: seq<'a3> * ?MultiY: seq<seq<'a3>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?XGap: int * ?YGap: int * ?Text: 'a4 * ?MultiText: seq<'a4> * ?ColorBar: ColorBar * ?ColorScale: Colorscale * ?ShowScale: bool * ?ReverseScale: bool * ?ZSmooth: SmoothAlg * ?Transpose: bool * ?UseWebGL: bool * ?ReverseYAxis: bool * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a3 :> IConvertible and 'a4 :> IConvertible) + 1 overload
...
static member Chart.Point: xy: seq<#System.IConvertible * #System.IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'c * ?MultiText: seq<'c> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'c :> System.IConvertible)
static member Chart.Point: x: seq<#System.IConvertible> * y: seq<#System.IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'c * ?MultiText: seq<'c> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'c :> System.IConvertible)
Multiple items
module List
from FSharp.Stats
<summary>
Module to compute common statistical measure on list
</summary>
--------------------
module List
from Microsoft.FSharp.Collections
<summary>Contains operations for working with values of type <see cref="T:Microsoft.FSharp.Collections.list`1" />.</summary>
<namespacedoc><summary>Operations for collections such as lists, arrays, sets, maps and sequences. See also
<a href="https://docs.microsoft.com/dotnet/fsharp/language-reference/fsharp-collection-types">F# Collection Types</a> in the F# Language Guide.
</summary></namespacedoc>
--------------------
type List =
new: unit -> List
static member geomspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float list
static member linspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float list
--------------------
type List<'T> =
| op_Nil
| op_ColonColon of Head: 'T * Tail: 'T list
interface IReadOnlyList<'T>
interface IReadOnlyCollection<'T>
interface IEnumerable
interface IEnumerable<'T>
member GetReverseIndex: rank: int * offset: int -> int
member GetSlice: startIndex: int option * endIndex: int option -> 'T list
static member Cons: head: 'T * tail: 'T list -> 'T list
member Head: 'T
member IsEmpty: bool
member Item: index: int -> 'T with get
...
<summary>The type of immutable singly-linked lists.</summary>
<remarks>Use the constructors <c>[]</c> and <c>::</c> (infix) to create values of this type, or
the notation <c>[1;2;3]</c>. Use the values in the <c>List</c> module to manipulate
values of this type, or pattern match against the values directly.
</remarks>
<exclude />
--------------------
new: unit -> List
val map: mapping: ('T -> 'U) -> list: 'T list -> 'U list
<summary>Builds a new collection whose elements are the results of applying the given function
to each of the elements of the collection.</summary>
<param name="mapping">The function to transform elements from the input list.</param>
<param name="list">The input list.</param>
<returns>The list of transformed elements.</returns>
<example id="map-1"><code lang="fsharp">
let inputs = [ "a"; "bbb"; "cc" ]
inputs |> List.map (fun x -> x.Length)
</code>
Evaluates to <c>[ 1; 3; 2 ]</c></example>
val x: float
static member Interpolation.predict: coef: InterpolationCoefficients -> (float -> float)
static member Chart.Line: xy: seq<#System.IConvertible * #System.IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'c * ?MultiText: seq<'c> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?LineColor: Color * ?LineColorScale: StyleParam.Colorscale * ?LineWidth: float * ?LineDash: StyleParam.DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?Fill: StyleParam.Fill * ?FillColor: Color * ?FillPattern: TraceObjects.Pattern * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'c :> System.IConvertible)
static member Chart.Line: x: seq<#System.IConvertible> * y: seq<#System.IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'c * ?MultiText: seq<'c> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?LineColor: Color * ?LineColorScale: StyleParam.Colorscale * ?LineWidth: float * ?LineDash: StyleParam.DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?Fill: StyleParam.Fill * ?FillColor: Color * ?FillPattern: TraceObjects.Pattern * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'c :> System.IConvertible)
static member Chart.withTraceInfo: ?Name: string * ?Visible: StyleParam.Visible * ?ShowLegend: bool * ?LegendRank: int * ?LegendGroup: string * ?LegendGroupTitle: Title -> (GenericChart.GenericChart -> GenericChart.GenericChart)
property Interpolation.Polynomial.PolynomialCoef.Predict: float -> float with get
<summary>
takes x value to predict the corresponding interpolating y value
</summary>
<param name="x">x value of which the corresponding y value should be predicted</param>
<returns>predicted y value with given polynomial coefficients at X=x</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|1.;2.;3.;4.;5.;6.|]
// e.g. temperature measured at noon of the days specified in xData
let yData = vector [|4.;7.;9.;8.;7.;9.;|]
// Estimate the polynomial coefficients. In Interpolation the order is equal to the data length - 1.
let coefficients =
Interpolation.Polynomial.coefficients xData yData
// Predict the temperature value at midnight between day 1 and 2.
coefficients.Predict 1.5
</code></example>
static member Chart.combine: gCharts: seq<GenericChart.GenericChart> -> GenericChart.GenericChart
static member Chart.withTemplate: template: Template -> (GenericChart.GenericChart -> GenericChart.GenericChart)
module ChartTemplates
from Plotly.NET
val lightMirrored: Template
static member Chart.withXAxisStyle: ?TitleText: string * ?TitleFont: Font * ?TitleStandoff: int * ?Title: Title * ?Color: Color * ?AxisType: StyleParam.AxisType * ?MinMax: (#System.IConvertible * #System.IConvertible) * ?Mirror: StyleParam.Mirror * ?ShowSpikes: bool * ?SpikeColor: Color * ?SpikeThickness: int * ?ShowLine: bool * ?LineColor: Color * ?ShowGrid: bool * ?GridColor: Color * ?GridDash: StyleParam.DrawingStyle * ?ZeroLine: bool * ?ZeroLineColor: Color * ?Anchor: StyleParam.LinearAxisId * ?Side: StyleParam.Side * ?Overlaying: StyleParam.LinearAxisId * ?Domain: (float * float) * ?Position: float * ?CategoryOrder: StyleParam.CategoryOrder * ?CategoryArray: seq<#System.IConvertible> * ?RangeSlider: LayoutObjects.RangeSlider * ?RangeSelector: LayoutObjects.RangeSelector * ?BackgroundColor: Color * ?ShowBackground: bool * ?Id: StyleParam.SubPlotId -> (GenericChart.GenericChart -> GenericChart.GenericChart)
static member Chart.withYAxisStyle: ?TitleText: string * ?TitleFont: Font * ?TitleStandoff: int * ?Title: Title * ?Color: Color * ?AxisType: StyleParam.AxisType * ?MinMax: (#System.IConvertible * #System.IConvertible) * ?Mirror: StyleParam.Mirror * ?ShowSpikes: bool * ?SpikeColor: Color * ?SpikeThickness: int * ?ShowLine: bool * ?LineColor: Color * ?ShowGrid: bool * ?GridColor: Color * ?GridDash: StyleParam.DrawingStyle * ?ZeroLine: bool * ?ZeroLineColor: Color * ?Anchor: StyleParam.LinearAxisId * ?Side: StyleParam.Side * ?Overlaying: StyleParam.LinearAxisId * ?AutoShift: bool * ?Shift: int * ?Domain: (float * float) * ?Position: float * ?CategoryOrder: StyleParam.CategoryOrder * ?CategoryArray: seq<#System.IConvertible> * ?RangeSlider: LayoutObjects.RangeSlider * ?RangeSelector: LayoutObjects.RangeSelector * ?BackgroundColor: Color * ?ShowBackground: bool * ?Id: StyleParam.SubPlotId -> (GenericChart.GenericChart -> GenericChart.GenericChart)
static member Chart.withSize: width: float * height: float -> (GenericChart.GenericChart -> GenericChart.GenericChart)
static member Chart.withSize: ?Width: int * ?Height: int -> (GenericChart.GenericChart -> GenericChart.GenericChart)
module GenericChart
from Plotly.NET
<summary>
Module to represent a GenericChart
</summary>
val toChartHTML: gChart: GenericChart.GenericChart -> string
val xData: Vector<float>
val yData: Vector<float>
val coefficients: Interpolation.Polynomial.PolynomialCoef
module Polynomial
from FSharp.Stats.InterpolationModule
<summary>
Calculates polynomials that interpolatethe two dimensional data. The polynomial order is equal to the number of data points - 1.
</summary>
<remarks>
In general a polynomial with degree = datapointNumber - 1 is flexible enough to interpolate all datapoints.
But polynomial regression with degree = datapointNumber - 1 cannot be used for polynomial interpolation
because the least squares approach is not sufficient to converge interpolating.
</remarks>
val interpolate: xData: Vector<float> -> yData: Vector<float> -> Interpolation.Polynomial.PolynomialCoef
<summary>
Calculates the polynomial coefficients for interpolating the given unsorted data.
</summary>
<remarks>No duplicates allowed!</remarks>
<param name="xData">Note: Must not contain duplicate x values (use Approximation.regularizeValues to preprocess data!)</param>
<param name="yData">vector of y values</param>
<returns>vector of polynomial coefficients sorted as [constant;linear;quadratic;...]</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|1.;2.;3.;4.;5.;6.|]
// e.g. e.g. temperature measured at noon of the days specified in xData
let yData = vector [|4.;7.;9.;8.;7.;9.;|]
// Estimate the polynomial coefficients. In Interpolation the order is equal to the data length - 1.
let coefficients =
Interpolation.Polynomial.coefficients xData yData
</code></example>
val interpolFunction: x: float -> float
val predict: coef: Interpolation.Polynomial.PolynomialCoef -> x: float -> float
<summary>
takes polynomial coefficients and x value to predict the corresponding interpolating y value
</summary>
<param name="coef">polynomial coefficients (e.g. determined by Polynomial.coefficients), sorted as [constant;linear;quadratic;...]</param>
<param name="x">x value of which the corresponding y value should be predicted</param>
<returns>predicted y value with given polynomial coefficients at X=x</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|1.;2.;3.;4.;5.;6.|]
// e.g. temperature measured at noon of the days specified in xData
let yData = vector [|4.;7.;9.;8.;7.;9.;|]
// Estimate the polynomial coefficients. In Interpolation the order is equal to the data length - 1.
let coefficients =
Interpolation.Polynomial.coefficients xData yData
// Predict the temperature value at midnight between day 1 and 2.
Interpolation.Polynomial.fit coefficients 1.5
</code></example>
val rawChart: GenericChart.GenericChart
val interpolPol: GenericChart.GenericChart
val fit: (float * float)[]
Multiple items
module Array
from FSharp.Stats
<summary>
Module to compute common statistical measure on array
</summary>
--------------------
module Array
from Microsoft.FSharp.Collections
<summary>Contains operations for working with arrays.</summary>
<remarks>
See also <a href="https://docs.microsoft.com/dotnet/fsharp/language-reference/arrays">F# Language Guide - Arrays</a>.
</remarks>
--------------------
type Array =
new: unit -> Array
static member geomspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float array
static member linspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float[]
--------------------
new: unit -> Array
val map: mapping: ('T -> 'U) -> array: 'T[] -> 'U[]
<summary>Builds a new array whose elements are the results of applying the given function
to each of the elements of the array.</summary>
<param name="mapping">The function to transform elements of the array.</param>
<param name="array">The input array.</param>
<returns>The array of transformed elements.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input array is null.</exception>
<example id="map-1"><code lang="fsharp">
let inputs = [| "a"; "bbb"; "cc" |]
inputs |> Array.map (fun x -> x.Length)
</code>
Evaluates to <c>[| 1; 3; 2 |]</c></example>
val chartPol: GenericChart.GenericChart
val xValues: Vector<float>
val yValues: Vector<float>
val coeffSpline: CubicSpline.CubicSplineCoef
val interpolate: boundaryCondition: CubicSpline.BoundaryCondition -> xValues: Vector<float> -> yValues: Vector<float> -> CubicSpline.CubicSplineCoef
<summary>
Computes coefficients for piecewise interpolating splines. In the form of [a0;b0;c0;d0;a1;b1;...;d(n-2)].
where: fn(x) = (an)x^3+(bn)x^2+(cn)x+(dn)
</summary>
<param name="boundaryCondition">Condition that defines how slopes and curvatures are defined at the left- and rightmost knot</param>
<param name="xValues">Note: Must not contain duplicate x values (use Approximation.regularizeValues to preprocess data!)</param>
<param name="yValues">function value at x values</param>
<returns>Coefficients that define the interpolating function.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.interpolate CubicSpline.BoundaryConditions.Natural xData yData
</code></example>
union case CubicSpline.BoundaryCondition.Natural: CubicSpline.BoundaryCondition
<summary>most used spline variant: f'' at borders is set to 0</summary>
val interpolateFunctionWithinRange: x: float -> float
val predictWithinRange: coefficients: CubicSpline.CubicSplineCoef -> x: float -> float
<summary>
Returns function that takes x value (that lies within the range of input x values) and predicts the corresponding interpolating y value.
</summary>
<param name="coefficients">Interpolation functions coefficients.</param>
<param name="x">X value of which the y value should be predicted.</param>
<returns>Function that takes an x value and returns function value.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.interpolate CubicSpline.BoundaryConditions.Natural xData yData
// get interpolating value
let func = CubicSpline.predictWithinRange(coefLinSpl)
// get function value at x=3.4
func 3.4
</code></example>
<remarks>Only defined within the range of the given xValues!</remarks>
val interpolateFunction: x: float -> float
val predict: coefficients: CubicSpline.CubicSplineCoef -> x: float -> float
<summary>
Returns function that takes x value and predicts the corresponding interpolating y value.
</summary>
<param name="coefficients">Interpolation functions coefficients.</param>
<param name="x">X value of which the y value should be predicted.</param>
<returns>Function that takes an x value and returns function value.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.interpolate CubicSpline.BoundaryConditions.Natural xData yData
// get interpolating function
let func = CubicSpline.predict(coefLinSpl)
// get function value at x=3.4
func 3.4
</code></example>
<remarks>x values outside of the xValue range are predicted by straight lines defined by the nearest knot!</remarks>
val coeffPolynomial: Polynomial.PolynomialCoef
val interpolate: xData: Vector<float> -> yData: Vector<float> -> Polynomial.PolynomialCoef
<summary>
Calculates the polynomial coefficients for interpolating the given unsorted data.
</summary>
<remarks>No duplicates allowed!</remarks>
<param name="xData">Note: Must not contain duplicate x values (use Approximation.regularizeValues to preprocess data!)</param>
<param name="yData">vector of y values</param>
<returns>vector of polynomial coefficients sorted as [constant;linear;quadratic;...]</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|1.;2.;3.;4.;5.;6.|]
// e.g. e.g. temperature measured at noon of the days specified in xData
let yData = vector [|4.;7.;9.;8.;7.;9.;|]
// Estimate the polynomial coefficients. In Interpolation the order is equal to the data length - 1.
let coefficients =
Interpolation.Polynomial.coefficients xData yData
</code></example>
val interpolateFunctionPol: x: float -> float
val predict: coef: Polynomial.PolynomialCoef -> x: float -> float
<summary>
takes polynomial coefficients and x value to predict the corresponding interpolating y value
</summary>
<param name="coef">polynomial coefficients (e.g. determined by Polynomial.coefficients), sorted as [constant;linear;quadratic;...]</param>
<param name="x">x value of which the corresponding y value should be predicted</param>
<returns>predicted y value with given polynomial coefficients at X=x</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|1.;2.;3.;4.;5.;6.|]
// e.g. temperature measured at noon of the days specified in xData
let yData = vector [|4.;7.;9.;8.;7.;9.;|]
// Estimate the polynomial coefficients. In Interpolation the order is equal to the data length - 1.
let coefficients =
Interpolation.Polynomial.coefficients xData yData
// Predict the temperature value at midnight between day 1 and 2.
Interpolation.Polynomial.fit coefficients 1.5
</code></example>
val coeffLinearSpline: LinearSpline.LinearSplineCoef
module LinearSpline
from FSharp.Stats.InterpolationModule
<summary>
Module to create linear splines from x,y coordinates. x,y coordinates are interpolated by straight lines between two knots.
</summary>
<remarks>Equivalent to interval-wise simple linear regression between any neighbouring pair of data.</remarks>
val interpolate: xData: float array -> yData: float array -> LinearSpline.LinearSplineCoef
<summary>
Returns the linear spline interpolation coefficients from unsorted x,y data.
</summary>
<param name="xData">Collection of x values.</param>
<param name="yData">Collection of y values.</param>
<returns>x-values, intersects, and slopes of interpolating lines</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get slopes and intersects for interpolating straight lines
let coefficients =
Interpolation.LinearSpline.interpolate xData yData
</code></example>
<remarks>Must not contain duplicate x values. Use Approximation.regularizeValues to preprocess data!</remarks>
val ofSeq: source: seq<'T> -> 'T[]
<summary>Builds a new array from the given enumerable object.</summary>
<param name="source">The input sequence.</param>
<returns>The array of elements from the sequence.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input sequence is null.</exception>
<example id="ofseq-1"><code lang="fsharp">
let inputs = seq { 1; 2; 5 }
inputs |> Array.ofSeq
</code>
Evaluates to <c>[| 1; 2; 5 |]</c>.
</example>
val interpolateFunctionLinSp: (float -> float)
val predict: lsc: LinearSpline.LinearSplineCoef -> x: float -> float
<summary>
Predicts the y value at point x. A straight line is fitted between the neighboring x values given.
</summary>
<param name="lsc">Linear spline coefficients given as input x values, intersects, and slopes.</param>
<param name="x">X value at which the corresponding y value should be predicted</param>
<returns>Y value corresponding to the given x value.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get slopes and intersects for interpolating straight lines
let coefficients =
Interpolation.LinearSpline.initInterpolate xData yData
// get y value at 3.4
Interpolation.LinearSpline.interpolate coefficients 3.4
</code></example>
<remarks>X values that don't not lie within the range of the input x values, are predicted using the nearest interpolation line!</remarks>
val splineChart: GenericChart.GenericChart
static member Chart.withLineStyle: ?BackOff: StyleParam.BackOff * ?AutoColorScale: bool * ?CAuto: bool * ?CMax: float * ?CMid: float * ?CMin: float * ?Color: Color * ?ColorAxis: StyleParam.SubPlotId * ?Colorscale: StyleParam.Colorscale * ?ReverseScale: bool * ?ShowScale: bool * ?ColorBar: ColorBar * ?Dash: StyleParam.DrawingStyle * ?Shape: StyleParam.Shape * ?Simplify: bool * ?Smoothing: float * ?Width: float * ?MultiWidth: seq<float> * ?OutlierColor: Color * ?OutlierWidth: float -> (GenericChart.GenericChart -> GenericChart.GenericChart)
module StyleParam
from Plotly.NET
type DrawingStyle =
| Solid
| Dash
| Dot
| DashDot
| LongDash
| LongDashDot
| User of seq<int>
member Convert: unit -> obj
override ToString: unit -> string
static member convert: (DrawingStyle -> obj)
static member toString: (DrawingStyle -> string)
<summary>
Dash: Sets the drawing style of the lines segments in this trace.
Sets the style of the lines. Set to a dash string type or a dash length in px.
</summary>
union case StyleParam.DrawingStyle.Dash: StyleParam.DrawingStyle
static member Chart.withTitle: title: Title -> (GenericChart.GenericChart -> GenericChart.GenericChart)
static member Chart.withTitle: title: string * ?TitleFont: Font -> (GenericChart.GenericChart -> GenericChart.GenericChart)
val derivativeChart: GenericChart.GenericChart
val getFirstDerivative: coefficients: CubicSpline.CubicSplineCoef -> x: float -> float
<summary>
Returns function that takes x value and predicts the corresponding slope.
</summary>
<param name="coefficients">Interpolation functions coefficients.</param>
<param name="x">X value of which the slope should be predicted.</param>
<returns>Function that takes an x value and returns the function slope.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.interpolate CubicSpline.BoundaryConditions.Natural xData yData
// get slope function
let func = CubicSpline.getFirstDerivative(coefLinSpl)
// get slope at x=3.4
func 3.4
</code></example>
<remarks>x values outside of the xValue range are predicted by straight lines defined by the nearest knot!</remarks>
val getSecondDerivative: coefficients: CubicSpline.CubicSplineCoef -> x: float -> float
<summary>
Returns function that takes x value and predicts the corresponding curvature.
</summary>
<param name="coefficients">Interpolation functions coefficients.</param>
<param name="x">X value of which the curvature should be predicted.</param>
<returns>Function that takes an x value and returns the function curvature.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.interpolate CubicSpline.BoundaryConditions.Natural xData yData
// get curvature function
let func = CubicSpline.getSecondDerivative(coefLinSpl)
// get curvature at x=3.4
func 3.4
</code></example>
<remarks>x values outside of the xValue range are predicted by straight lines defined by the nearest knot!</remarks>
val getThirdDerivative: coefficients: CubicSpline.CubicSplineCoef -> x: float -> float
<summary>
Returns function that takes x value and predicts the corresponding third derivative.
</summary>
<param name="coefficients">Interpolation functions coefficients.</param>
<param name="x">X value of which the y value should be predicted.</param>
<returns>Function that takes an x value and returns the function third derivative.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.interpolate CubicSpline.BoundaryConditions.Natural xData yData
// get third derivative function
let func = CubicSpline.getThirdDerivative(coefLinSpl)
// get third derivative at x=3.4
func 3.4
</code></example>
<remarks>x values outside of the xValue range are predicted by straight lines defined by the nearest knot!</remarks>
val xVal: float[]
val yVal: float[]
val akimaCoeffs: Akima.SubSplineCoef
module Akima
from FSharp.Stats.InterpolationModule
<summary>
Module to create piecewise cubic polynomials (cubic subsplines) from x,y coordinates.
Akima subsplines are more flexible than standard cubic splines because the are NOT continuous in the function curvature, thereby diminishing oscillating behaviour.
</summary>
val interpolate: xValues: float[] -> yValues: float[] -> Akima.SubSplineCoef
<summary>
Computes coefficients for piecewise interpolating subsplines.
</summary>
<param name="xValues">Note: Must not contain duplicate x values (use Approximation.regularizeValues to preprocess data!)</param>
<param name="yValues">function value at x values</param>
<returns>Coefficients that define the interpolating function.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
Akima.interpolate xData yData
</code></example>
<remarks>Second derivative (curvature) is NOT continuous at knots to allow higher flexibility to reduce oscillations! For reference see: http://www.dorn.org/uni/sls/kap06/f08_0204.htm.</remarks>
val akima: GenericChart.GenericChart
val predict: splineCoeffs: Akima.SubSplineCoef -> xVal: float -> float
<summary>
Returns function that takes x value and predicts the corresponding interpolating y value.
</summary>
<param name="splineCoeffs">Interpolation functions coefficients.</param>
<param name="xVal">X value of which the y value should be predicted.</param>
<returns>Function that takes an x value and returns function value.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
Akima.interpolate xData yData
// get function to predict y value
let interpolFunc =
Akima.predict coefficients
// get function value at x=3.4
interpolFunc 3.4
</code></example>
<remarks>Second derivative (curvature) is NOT continuous at knots to allow higher flexibility to reduce oscillations! For reference see: http://www.dorn.org/uni/sls/kap06/f08_0204.htm.</remarks>
val cubicCoeffs: CubicSpline.CubicSplineCoef
val cubicSpline: GenericChart.GenericChart
val akimaChart: GenericChart.GenericChart
val xDataH: Vector<float>
val yDataH: Vector<float>
val tryMonotoneSlope: CubicSpline.Hermite.HermiteCoef
module Hermite
from FSharp.Stats.InterpolationModule.CubicSpline
<summary>
Hermite cubic splines are defined by the function values and their slopes (first derivatives). If the slopws are unknown, they must be estimated.
</summary>
val interpolatePreserveMonotonicity: xData: Vector<float> -> yData: Vector<float> -> CubicSpline.Hermite.HermiteCoef
<summary>
Computes coefficients for piecewise interpolating splines.
If the knots are monotone in/decreasing, the spline also is monotone (CJC Kruger method)
The x data has to be sorted ascending
</summary>
<param name="yData">function value at x values</param>
<returns>Coefficients that define the interpolating function.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;6.;13.;13.1|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.Hermite.interpolatePreserveMonotonicity xData yData
</code></example>
<remarks>Second derivative (curvature) is NOT necessarily continuous at knots to allow higher flexibility to reduce oscillations!</remarks>
<remarks>Constrained Cubic Spline Interpolation for Chemical Engineering Applications by CJC Kruger </remarks>
val funHermite: x: float -> float
val predict: coef: CubicSpline.Hermite.HermiteCoef -> x: float -> float
<summary>
Returns function that takes x value and predicts the corresponding interpolating y value.
</summary>
<param name="coefficients">Interpolation functions coefficients.</param>
<param name="x">X value of which the y value should be predicted.</param>
<returns>Function that takes an x value and returns function value.</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|0.;1.;5.;4.;3.;|]
// some measured feature
let yData = vector [|1.;5.;4.;13.;17.|]
// get coefficients for piecewise interpolating cubic polynomials
let coefficients =
CubicSpline.Hermite.interpolate xData yData
// get interpolating function
let func = CubicSpline.Hermite.predict coefLinSpl
// get function value at x=3.4
func 3.4
</code></example>
<remarks>x values outside of the xValue range are predicted by straight lines defined by the nearest knot!</remarks>
val coeffSpl: CubicSpline.CubicSplineCoef
val funNaturalSpline: x: float -> float
val coeffPolInterpol: Polynomial.PolynomialCoef
val funPolInterpol: x: float -> float
val splineComparison: GenericChart.GenericChart
val bezierInterpolation: GenericChart.GenericChart
val t: float
val p0: Vector<float>
val c0: Vector<float>
val c1: Vector<float>
val c2: Vector<float>
val c3: Vector<float>
val p1: Vector<float>
val toPoint: (vector -> float * float)
val v: vector
val interpolate: (float -> float * float)
module Bezier
from FSharp.Stats.InterpolationModule
<summary>
Bezier interpolates data between 2 points using a number of control points, which determine the degree of the interpolated curve.
</summary>
val interpolate: values: vector[] -> (float -> vector)
<summary>
This implements Bezier interpolation using De Casteljau's algorithm.
</summary>
<param name="values">an array containg the starting point, the control points, and the end point to use for interpolation</param>
<returns>The generated interpolation function</returns>
type Color =
override Equals: other: obj -> bool
override GetHashCode: unit -> int
static member fromARGB: a: int -> r: int -> g: int -> b: int -> Color
static member fromColorScaleValues: c: seq<#IConvertible> -> Color
static member fromColors: c: seq<Color> -> Color
static member fromHex: s: string -> Color
static member fromKeyword: c: ColorKeyword -> Color
static member fromRGB: r: int -> g: int -> b: int -> Color
static member fromString: c: string -> Color
member Value: obj
<summary>
Plotly color can be a single color, a sequence of colors, or a sequence of numeric values referencing the color of the colorscale obj
</summary>
static member Color.fromHex: s: string -> Color
static member Chart.withMarkerStyle: ?Angle: float * ?AngleRef: StyleParam.AngleRef * ?AutoColorScale: bool * ?CAuto: bool * ?CMax: float * ?CMid: float * ?CMin: float * ?Color: Color * ?Colors: seq<Color> * ?ColorAxis: StyleParam.SubPlotId * ?ColorBar: ColorBar * ?Colorscale: StyleParam.Colorscale * ?CornerRadius: int * ?Gradient: TraceObjects.Gradient * ?Outline: Line * ?MaxDisplayed: int * ?Opacity: float * ?MultiOpacity: seq<float> * ?Pattern: TraceObjects.Pattern * ?ReverseScale: bool * ?ShowScale: bool * ?Size: int * ?MultiSize: seq<int> * ?SizeMin: int * ?SizeMode: StyleParam.MarkerSizeMode * ?SizeRef: int * ?StandOff: float * ?MultiStandOff: seq<float> * ?Symbol: StyleParam.MarkerSymbol * ?MultiSymbol: seq<StyleParam.MarkerSymbol> * ?Symbol3D: StyleParam.MarkerSymbol3D * ?MultiSymbol3D: seq<StyleParam.MarkerSymbol3D> * ?OutlierColor: Color * ?OutlierWidth: int -> (GenericChart.GenericChart -> GenericChart.GenericChart)
val bezierInterpolation3d: GenericChart.GenericChart
val to3Dpoint: (vector -> float * float * float)
val interpolate: (float -> float * float * float)
static member Chart.Point3D: xyz: seq<#System.IConvertible * #System.IConvertible * #System.IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a3 * ?MultiText: seq<'a3> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol3D * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol3D> * ?Marker: TraceObjects.Marker * ?CameraProjectionType: StyleParam.CameraProjectionType * ?Camera: LayoutObjects.Camera * ?Projection: TraceObjects.Projection * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'a3 :> System.IConvertible)
static member Chart.Point3D: x: seq<#System.IConvertible> * y: seq<#System.IConvertible> * z: seq<#System.IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'd * ?MultiText: seq<'d> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol3D * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol3D> * ?Marker: TraceObjects.Marker * ?CameraProjectionType: StyleParam.CameraProjectionType * ?Camera: LayoutObjects.Camera * ?Projection: TraceObjects.Projection * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'd :> System.IConvertible)
static member Chart.Line3D: xyz: seq<#System.IConvertible * #System.IConvertible * #System.IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'd * ?MultiText: seq<'d> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol3D * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol3D> * ?Marker: TraceObjects.Marker * ?LineColor: Color * ?LineColorScale: StyleParam.Colorscale * ?LineWidth: float * ?LineDash: StyleParam.DrawingStyle * ?Line: Line * ?CameraProjectionType: StyleParam.CameraProjectionType * ?Camera: LayoutObjects.Camera * ?Projection: TraceObjects.Projection * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'd :> System.IConvertible)
static member Chart.Line3D: x: seq<#System.IConvertible> * y: seq<#System.IConvertible> * z: seq<#System.IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a3 * ?MultiText: seq<'a3> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol3D * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol3D> * ?Marker: TraceObjects.Marker * ?LineColor: Color * ?LineColorScale: StyleParam.Colorscale * ?LineWidth: float * ?LineDash: StyleParam.DrawingStyle * ?Line: Line * ?CameraProjectionType: StyleParam.CameraProjectionType * ?Camera: LayoutObjects.Camera * ?Projection: TraceObjects.Projection * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'a3 :> System.IConvertible)
val xs: float[]
val ys: float[]
val chebyChart: GenericChart.GenericChart
val coeffs: Polynomial.PolynomialCoef
val interpolatingFunction: x: float -> float
val interpolChart: GenericChart.GenericChart
val ys_interpol: seq<float * float>
Multiple items
module Seq
from FSharp.Stats
<summary>
Module to compute common statistical measure
</summary>
--------------------
module Seq
from Microsoft.FSharp.Collections
<summary>Contains operations for working with values of type <see cref="T:Microsoft.FSharp.Collections.seq`1" />.</summary>
--------------------
type Seq =
new: unit -> Seq
static member geomspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> seq<float>
static member linspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> seq<float>
--------------------
new: unit -> Seq
val map: mapping: ('T -> 'U) -> source: seq<'T> -> seq<'U>
<summary>Builds a new collection whose elements are the results of applying the given function
to each of the elements of the collection. The given function will be applied
as elements are demanded using the <c>MoveNext</c> method on enumerators retrieved from the
object.</summary>
<remarks>The returned sequence may be passed between threads safely. However,
individual IEnumerator values generated from the returned sequence should not be accessed concurrently.</remarks>
<param name="mapping">A function to transform items from the input sequence.</param>
<param name="source">The input sequence.</param>
<returns>The result sequence.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input sequence is null.</exception>
<example id="item-1"><code lang="fsharp">
let inputs = ["a"; "bbb"; "cc"]
inputs |> Seq.map (fun x -> x.Length)
</code>
Evaluates to a sequence yielding the same results as <c>seq { 1; 3; 2 }</c></example>
val cbChart: GenericChart.GenericChart
val xs_cheby: Vector<float>
Multiple items
module Approximation
from FSharp.Stats.InterpolationModule
<summary>
Approximation
</summary>
--------------------
type Approximation =
new: unit -> Approximation
static member approxWithPolynomial: f: (float -> float) * i: Interval<float> * n: int * spacing: Spacing -> PolynomialCoef
static member approxWithPolynomialFromValues: xData: seq<float> * yData: seq<float> * n: int * spacing: Spacing -> PolynomialCoef
--------------------
new: unit -> Approximation
val chebyshevNodes: interval: Interval<float> -> n: int -> Vector<float>
<summary>
Creates a collection of ordered x values within a given interval. The spacing is according to Chebyshev to remove runges phenomenon when approximating a given function.
</summary>
<param name="interval">start and end value of interpolation range</param>
<param name="n">number of points that should be placed within the interval (incl. start and end)</param>
<returns>Collection of chebyshev spaced x values.</returns>
<remarks>www.mscroggs.co.uk/blog/57</remarks>
Multiple items
module Interval
from FSharp.Stats
--------------------
type Interval<'a (requires comparison)> =
| Closed of 'a * 'a
| LeftOpen of 'a * 'a
| RightOpen of 'a * 'a
| Open of 'a * 'a
| Empty
member GetEnd: unit -> 'a
member GetStart: unit -> 'a
override ToString: unit -> string
member ToTuple: unit -> 'a * 'a
member liesInInterval: value: 'a -> bool
static member CreateClosed: min: 'b * max: 'b -> Interval<'b> (requires comparison)
static member CreateLeftOpen: min: 'a1 * max: 'a1 -> Interval<'a1> (requires comparison)
static member CreateOpen: min: 'c * max: 'c -> Interval<'c> (requires comparison)
static member CreateRightOpen: min: 'a0 * max: 'a0 -> Interval<'a0> (requires comparison)
static member ofSeq: source: seq<'a> -> Interval<'a>
...
<summary>
Closed interval [Start,End]
</summary>
static member Interval.CreateClosed: min: 'b * max: 'b -> Interval<'b> (requires comparison)
Multiple items
val float: value: 'T -> float (requires member op_Explicit)
<summary>Converts the argument to 64-bit float. This is a direct conversion for all
primitive numeric types. For strings, the input is converted using <c>Double.Parse()</c>
with InvariantCulture settings. Otherwise the operation requires an appropriate
static conversion method on the input type.</summary>
<param name="value">The input value.</param>
<returns>The converted float</returns>
<example id="float-example"><code lang="fsharp"></code></example>
--------------------
[<Struct>]
type float = System.Double
<summary>An abbreviation for the CLI type <see cref="T:System.Double" />.</summary>
<category>Basic Types</category>
--------------------
type float<'Measure> =
float
<summary>The type of double-precision floating point numbers, annotated with a unit of measure.
The unit of measure is erased in compiled code and when values of this type
are analyzed using reflection. The type is representationally equivalent to
<see cref="T:System.Double" />.</summary>
<category index="6">Basic Types with Units of Measure</category>
val ys_cheby: Vector<float>
val ls: LinearSpline.LinearSplineCoef
Multiple items
module Vector
from FSharp.Stats
--------------------
type Vector<'T> =
interface IEnumerable
interface IEnumerable<'T>
interface IStructuralEquatable
interface IStructuralComparable
interface IComparable
new: opsV: INumeric<'T> option * arrV: 'T array -> Vector<'T>
override Equals: yobj: obj -> bool
override GetHashCode: unit -> int
member GetSlice: start: int option * finish: int option -> Vector<'T>
member Permute: p: permutation -> Vector<'T>
...
--------------------
new: opsV: INumeric<'T> option * arrV: 'T array -> Vector<'T>
val map: mapping: (float -> float) -> vector: vector -> Vector<float>
<summary>Builds a new vector whose elements are the results of applying the given function to each of the elements of the vector.</summary>
<remarks></remarks>
<param name="mapping"></param>
<param name="vector"></param>
<returns></returns>
<example><code></code></example>
val coeffs_cheby: Polynomial.PolynomialCoef
static member Approximation.approxWithPolynomialFromValues: xData: seq<float> * yData: seq<float> * n: int * spacing: Approximation.Spacing -> Polynomial.PolynomialCoef
argument n: int
<summary>
Determines polynomial coefficients to approximate the given data with (i) n equally spaced nodes or (ii) n nodes spaced according to Chebyshev.
Use Polynomial.fit to get a polynomial function of the coefficients.
</summary>
<param name="xData">Note: Must not contain duplicate x values (use Approximation.regularizeValues to preprocess data!)</param>
<param name="yData">vector of y values</param>
<param name="n">number of points that should be placed within the interval (incl. start and end)</param>
<param name="spacing">X values can be spaced equally or according to chebyshev.</param>
<returns>Coefficients for polynomial interpolation. Use Polynomial.fit to predict function values.</returns>
union case Approximation.Spacing.Chebyshev: Approximation.Spacing
val interpolating_cheby: x: float -> float
val interpolChart_cheby: GenericChart.GenericChart
val ys_interpol_cheby: seq<float * float>
val cbChart_cheby: GenericChart.GenericChart