
Summary: this tutorial demonstrates variou way to model growth curves, a commong task in any (micro)biological lab
Growth and other physiological parameters like size/weight/length can be modeled as function of time.
Several growth curve models have been proposed. Some of them are covered in this documentation.
For growth curve analysis the cell count data must be considered in log space. The exponential phase (log phase) then becomes linear.
After modeling, all growth parameters (maximal cell count, lag phase duration, and growth rate) can be derived from the model, so there is no
need for manual labelling of separate growth phases.
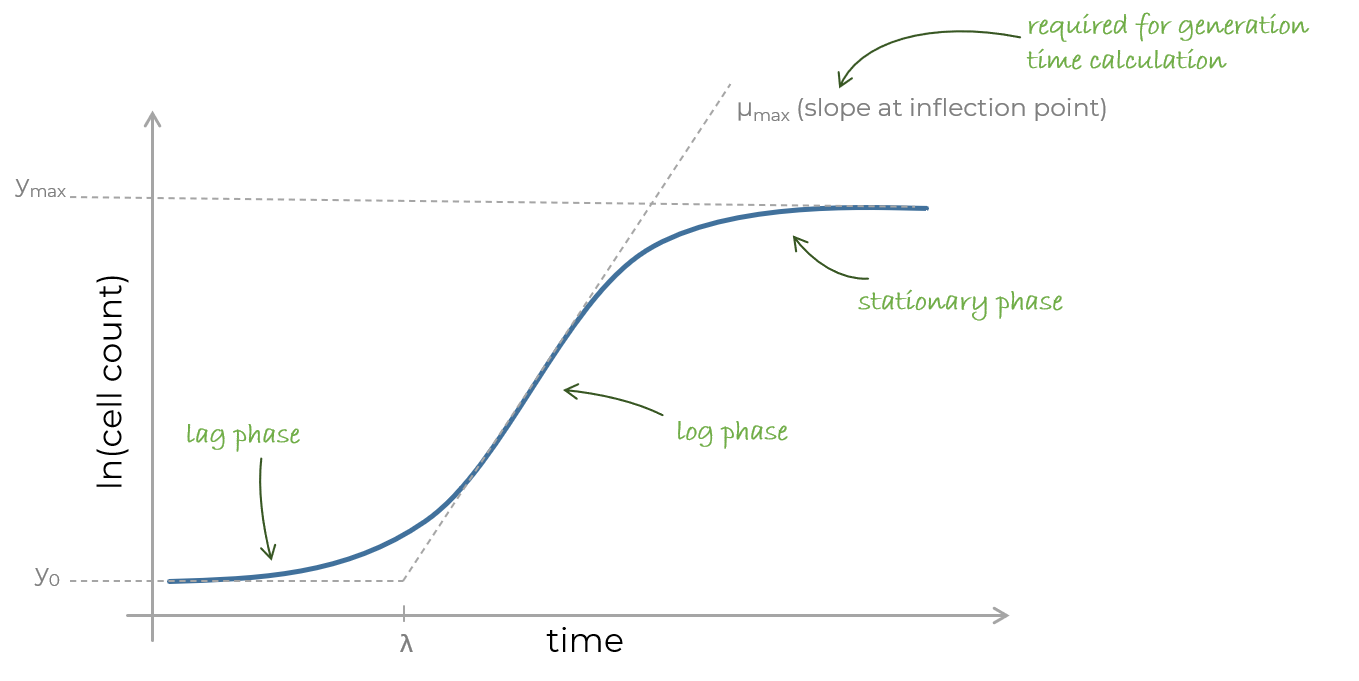
If specific parameters should be constrained to the users choice (like upper or lower asymptote), a constrained version of the
Levenberg-Marquardt solver can be used (LevenbergMarquardtConstrained
)! Accordingly, minimal and maximal parameter vectors must be provided.
open System
open FSharp.Stats
open FSharp.Stats.Fitting.NonLinearRegression
open FSharp.Stats.Fitting.LinearRegression
let time = [|0. .. 0.5 .. 8.|]
let cellCount =
[|
17000000.;16500000.;11000000.;14000000.;27000000.;40000000.;120000000.;
300000000.;450000000.;1200000000.;2700000000.;5000000000.;
8000000000.;11700000000.;12000000000.;13000000000.;12800000000.
|]
let cellCountLn = cellCount |> Array.map log
open Plotly.NET
let chartOrig =
Chart.Point(time,cellCount)
|> Chart.withTraceInfo "original data"
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withYAxisStyle "cells/ml"
let chartLog =
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
let growthChart =
[chartOrig;chartLog] |> Chart.Grid(2,1)
If growth phases are labelled manually, the exponential phase can be fitted with a regression line.
To determine the generation time, it is necessary to find the time interval it takes to double the count data.
When a log2 transform is used, a doubling of the original counts is achieved, when the log value moves 1 unit.
Keeping that in mind, the slope can be used to calculate the time it takes for the log2 data to increase 1 unit.
- slope * generation time = 1
- generation time = 1/slope
If a different log transform was used, the correction factor for the numerator is logx(2).
// The exponential phase was defined to be between time point 1.5 and 5.5.
let logPhaseX = vector time.[3..11]
let logPhaseY = vector cellCountLn.[3..11]
// regression coefficients as [intercept;slope]
let regressionCoeffs =
OLS.Linear.Univariable.fit logPhaseX logPhaseY
Here is a pre-evaluated version (to save time during the build process, as the solver takes quite some time.)
// The generation time is calculated by dividing log_x 2. by the regression line slope.
// The log transform must match the used data transform.
let slope = regressionCoeffs.[1]
let generationTime = log(2.) / slope
let fittedValues =
let f = OLS.Linear.Univariable.predict (Coefficients (vector [14.03859475; 1.515073487]))
logPhaseX |> Seq.map (fun x -> x,f x)
let chartLinearRegression =
[
Chart.Point(time,cellCountLn) |> Chart.withTraceInfo "log counts"
Chart.Line(fittedValues) |> Chart.withTraceInfo "fit"
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
let generationTimeManual = sprintf "The generation time (manual selection) is: %.1f min" ((log(2.)/1.5150)* 60.)
generationTimeManual
The generation time (manual selection) is: 27.5 min
In the following example the four parameter gompertz function is applied to cell count data (Gibson et al., 1988).
The Gompertz model is fitted using the Levenberg Marquardt solver. Initial parameters are estimated from the original data.
The expected generation time has to be approximated as initial guess.
For parameter interpretation the applied log transform is important and must be provided.
If other parameters than cell count (e.g. size or length) should be analyzed, use id
as value transform.
Gompertz parameters:
- A: lower asymptote
- B: relative growth rate (approximated by generation time consideration)
- C: upper asymptote - lower asymptote
- M: time point of inflection (maximal growth rate)
// The Levenberg Marquardt algorithm identifies the parameters that leads to the best fit
// of the gompertz models to the count data. The solver must be provided with initial parameters
// that are estimated in the following:
let solverOptions (xData :float []) (yDataLog :float []) expectedGenerationTime (usedLogTransform: float -> float) =
// lower asymptote
let a = Seq.min yDataLog
// upper asymptote - lower asymptote (y range)
let c = (Seq.max yDataLog) - a
// relative growth rate
let b = usedLogTransform 2. * Math.E / (expectedGenerationTime * c)
// time point of inflection (in gompertz model at f(x)=36% of the y range)
let m =
let yAtInflection = a + c * 0.36
Seq.zip xData yDataLog
|> Seq.minBy (fun (xValue,yValue) ->
Math.Abs (yValue - yAtInflection)
)
|> fst
createSolverOption 0.001 0.001 10000 [|a;b;c;m|]
// By solving the nonlinear fitting problem, the optimal model parameters are determined
let gompertzParams =
LevenbergMarquardt.estimatedParams
Table.GrowthModels.gompertz
(solverOptions time cellCountLn 1. log)
0.1
10.
time
cellCountLn
let fittingFunction =
Table.GrowthModels.gompertz.GetFunctionValue gompertzParams
let fittedValuesGompertz =
// The parameter were determined locally for saving time during build processes
//let f = Table.GrowthModels.gompertz.GetFunctionValue (vector [|16.46850199; 0.7014917539; 7.274139441; 3.3947717|])
[time.[0] .. 0.1 .. Seq.last time]
|> Seq.map (fun x ->
x,fittingFunction x
)
|> Chart.Line
|> Chart.withTraceInfo "gompertz"
let fittedChartGompertz =
[
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
fittedValuesGompertz
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
The generation time can be calculated by dividing log(2) by the slope of the inflection point. The used log transform must
match the used log transform applied to the count data.
The four parameter Gompertz model allows the determination of generation times from its parameters (Gibson et al., 1988).
let generationtime (parametervector:vector) (logTransform:float -> float) =
logTransform 2. * Math.E / (parametervector.[1] * parametervector.[2])
let lag (parametervector:vector) =
(parametervector.[3] - 1.) / parametervector.[1]
let g = sprintf "The generation time (Gompertz) is: %.1f min" (60. * (generationtime gompertzParams log))
let l = sprintf "The lag phase duration is %.2f h" (lag gompertzParams)
"The generation time (Gompertz) is: 22.2 min"
|
"The lag phase duration is 3.41 h"
|
In the following other growth models are applied to the given data set:
- Richards
- Weibull
- Janoschek
- Exponential
- Verhulst
- Morgan-Mercer-Flodin
- von Bertalanffy
To determine the generation time, the slope at the inflection point must be calculated.
As explained above, the generation time can be calculated by: logx(2)/(slope at inflection) where x is the used
log transform.
Choose a appropriate growth model according to your needs.
For an overview please scroll down to see a combined diagram of all growth models.
Parameters:
- l: upper asymptote
- k: growth rate
- y: inflection point (x value)
- d: influences the inflection point on the y axis
let richardsParams =
LevenbergMarquardt.estimatedParams
Table.GrowthModels.richards
(createSolverOption 0.001 0.001 10000 [|23.;1.;3.4;2.|])
0.1
10.
time
cellCountLn
let fittingFunctionRichards =
Table.GrowthModels.richards.GetFunctionValue richardsParams
Here is a pre-evaluated version (to save time during the build process, as the solver takes quite some time.)
let generationtimeRichards (richardParameters:vector) =
let l = richardParameters.[0]
let k = richardParameters.[1]
let y = richardParameters.[2] //x value of inflection point
let d = richardParameters.[3]
let gradientFunctionRichards t =
(k*l*((d-1.)*Math.Exp(-k*(t-y))+1.)**(1./(1.-d)))/(Math.Exp(k*(t-y))+d-1.)
let maximalSlope =
gradientFunctionRichards y
log(2.) / maximalSlope
let fittedValuesRichards =
// The parameter were determined locally for saving time during build processes
let f = Table.GrowthModels.richards.GetFunctionValue (vector [|23.25211263; 7.053516315; 5.646889803; 111.0132522|])
[time.[0] .. 0.1 .. Seq.last time]
|> Seq.map (fun x ->
x,f x
)
|> Chart.Line
let fittedChartRichards =
fittedValuesRichards
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTraceInfo "richards"
let fittedChartRichardsS =
[
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
fittedValuesRichards
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTitle "richards"
let generationRichards = sprintf "The generation time (Richards) is: %.1f min" (generationtimeRichards (vector [|23.25211263; 7.053516315; 5.646889803; 111.0132522|]) * 60.)
"The generation time (Richards) is: 29.4 min"
|
Parameters:
- b: lower asymptote
- l: upper asymptote
- k: growth rate
- d: influences the inflection point position
let weibullParams =
LevenbergMarquardt.estimatedParams
Table.GrowthModels.weibull
(createSolverOption 0.001 0.001 10000 [|15.;25.;1.;5.|])
0.1
10.
time
cellCountLn
let fittingFunctionWeibull =
Table.GrowthModels.weibull.GetFunctionValue weibullParams
Here is a pre-evaluated version (to save time during the build process, as the solver takes quite some time.)
let generationtimeWeibull (weibullParameters:vector) =
let b = weibullParameters.[0]
let l = weibullParameters.[1]
let k = weibullParameters.[2]
let d = weibullParameters.[3]
let gradientFunctionWeibull t =
(d*(l-b)*(k*t)**d*Math.Exp(-((k*t)**d)))/t
let inflectionPointXValue =
(1./k)*((d-1.)/d)**(1./d)
let maximalSlope =
gradientFunctionWeibull inflectionPointXValue
log(2.) / maximalSlope
let fittedValuesWeibull =
// The parameter were determined locally for saving time during build processes
let f = Table.GrowthModels.weibull.GetFunctionValue (vector [|16.40632433; 23.35537293; 0.2277752116; 2.900806071|])
[time.[0] .. 0.1 .. Seq.last time]
|> Seq.map (fun x ->
x,f x
)
|> Chart.Line
let fittedChartWeibull =
fittedValuesWeibull
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTraceInfo "weibull"
let fittedChartWeibullS =
[
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
fittedValuesWeibull
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTitle "weibull"
let generationWeibull =
sprintf "The generation time (Weibull) is: %.1f min" (generationtimeWeibull (vector [|16.40632433; 23.35537293; 0.2277752116; 2.900806071|]) * 60.)
"The generation time (Weibull) is: 23.0 min"
|
Parameters:
- b: lower asymptote
- l: upper asymptote
- k: growth rate
- d: influences the inflection point position on the x axis
let janoschekParams =
LevenbergMarquardt.estimatedParams
Table.GrowthModels.janoschek
(createSolverOption 0.001 0.001 10000 [|15.;25.;1.;5.|])
0.1
10.
time
cellCountLn
let fittingFunctionJanoschek =
Table.GrowthModels.janoschek.GetFunctionValue janoschekParams
Here is a pre-evaluated version (to save time during the build process, as the solver takes quite some time.)
let generationtimeJanoschek (janoschekParameters:vector) =
let b = janoschekParameters.[0]
let l = janoschekParameters.[1]
let k = janoschekParameters.[2]
let d = janoschekParameters.[3]
let gradientFunctionJanoschek t =
d*k*(l-b)*t**(d-1.)*Math.Exp(-k*t**d)
//Chart to estimate point of maximal slope (inflection point)
let slopeChart() =
[time.[0] .. 0.1 .. 8.] |> List.map (fun x -> x, gradientFunctionJanoschek x) |> Chart.Line
let inflectionPointXValue =
3.795
let maximalSlope =
gradientFunctionJanoschek inflectionPointXValue
log(2.) / maximalSlope
let fittedValuesJanoschek =
// The parameter were determined locally for saving time during build processes
let f = Table.GrowthModels.janoschek.GetFunctionValue (vector [|16.40633962; 23.35535182; 0.01368422994; 2.900857027|])
[time.[0] .. 0.1 .. Seq.last time]
|> Seq.map (fun x ->
x,f x
)
|> Chart.Line
let fittedChartJanoschek =
fittedValuesJanoschek
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTraceInfo "janoschek"
let fittedChartJanoschekS =
[
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
fittedChartJanoschek
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTitle "janoschek"
let generationJanoschek =
sprintf "The generation time (Janoschek) is: %.1f min" (generationtimeJanoschek (vector [|16.40633962; 23.35535182; 0.01368422994; 2.900857027|]) * 60.)
"The generation time (Janoschek) is: 23.0 min"
|
The exponential model of course can not be applied to the lag phase.
Parameters:
- b: lower asymptote
- l: upper asymptote
- k: growth rate
let exponentialParams =
LevenbergMarquardt.estimatedParams
Table.GrowthModels.exponential
(createSolverOption 0.001 0.001 10000 [|15.;25.;0.5|])
0.1
10.
time.[6..]
cellCountLn.[6..]
let fittingFunctionExponential =
Table.GrowthModels.exponential.GetFunctionValue exponentialParams
Here is a pre-evaluated version (to save time during the build process, as the solver takes quite some time.)
let generationtimeExponential (expParameters:vector) =
let b = expParameters.[0]
let l = expParameters.[1]
let k = expParameters.[2]
let gradientFunctionExponential t =
k*(l-b)*Math.Exp(-k*t)
let maximalSlope =
gradientFunctionExponential time.[6]
log(2.) / maximalSlope
let fittedValuesExp =
// The parameter were determined locally for saving time during build processes
let f = Table.GrowthModels.exponential.GetFunctionValue (vector [|4.813988967; 24.39950361; 0.3939132175|])
[3.0 .. 0.1 .. Seq.last time]
|> Seq.map (fun x ->
x,f x
)
|> Chart.Line
let fittedChartExp =
fittedValuesExp
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTraceInfo "exponential"
let fittedChartExpS =
[
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
fittedChartExp
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTitle "exponential"
let generationExponential =
sprintf "The generation time (Exp) is: %.1f min" (generationtimeExponential (vector [|4.813988967; 24.39950361; 0.3939132175|]) * 60.)
"The generation time (Exp) is: 17.6 min"
|
The verhulst growth model is a logistic function with a lower asymptote fixed at y=0. A 4 parameter version allows
the lower asymptote to vary from 0.
Note: symmetric with inflection point at 50 % of y axis range
Parameters:
- l: upper asymptote
- k: x value at inflection point
- d: steepness
- b: lower asymptote
To apply the 3 parameter verhulst model with a fixed lower asymptote = 0 use the 'verhulst' model instead of 'verhulst4Param'.
let verhulstParams =
LevenbergMarquardt.estimatedParams
Table.GrowthModels.verhulst4Param
(createSolverOption 0.001 0.001 10000 [|25.;3.5;1.;15.|])
0.1
10.
time
cellCountLn
let fittingFunctionVerhulst() =
Table.GrowthModels.verhulst.GetFunctionValue verhulstParams
Here is a pre-evaluated version (to save time during the build process, as the solver takes quite some time.)
let generationtimeVerhulst (verhulstParameters:vector) =
let lmax = verhulstParameters.[0]
let k = verhulstParameters.[1]
let d = verhulstParameters.[2]
let lmin = verhulstParameters.[3]
let gradientFunctionVerhulst t =
((lmax-lmin)*Math.Exp((k-t)/d))/(d*(Math.Exp((k-t)/d)+1.)**2.)
let maximalSlope =
gradientFunctionVerhulst k
log(2.) / maximalSlope
let fittedValuesVerhulst =
// The parameter were determined locally for saving time during build processes
let f = Table.GrowthModels.verhulst4Param.GetFunctionValue (vector [|23.39504328; 3.577488116; 1.072136278; 15.77380824|])
[time.[0] .. 0.1 .. Seq.last time]
|> Seq.map (fun x ->
x,f x
)
|> Chart.Line
let fittedChartVerhulst =
fittedValuesVerhulst
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTraceInfo "verhulst"
let fittedChartVerhulstS =
[
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
fittedChartVerhulst
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTitle "verhulst"
let generationVerhulst =
sprintf "The generation time (Verhulst) is: %.1f min" (generationtimeVerhulst (vector [|23.39504328; 3.577488116; 1.072136278; 15.77380824|]) * 60.)
"The generation time (Verhulst) is: 23.4 min"
|
Parameters:
- b: count at t0
- l: upper asymptote
- k: growth rate
- d: influences the inflection point position
let morganMercerFlodinParams =
LevenbergMarquardt.estimatedParams
Table.GrowthModels.morganMercerFlodin
(createSolverOption 0.001 0.001 10000 [|15.;25.;0.2;3.|])
0.1
10.
time
cellCountLn
let fittingFunctionMMF() =
Table.GrowthModels.morganMercerFlodin.GetFunctionValue morganMercerFlodinParams
Here is a pre-evaluated version (to save time during the build process, as the solver takes quite some time.)
let generationtimeMmf (mmfParameters:vector) =
let b = mmfParameters.[0]
let l = mmfParameters.[1]
let k = mmfParameters.[2]
let d = mmfParameters.[3]
let gradientFunctionMmf t =
(d*(l-b)*(k*t)**d)/(t*((k*t)**d+1.)**2.)
//Chart to estimate point of maximal slope (inflection point)
let slopeChart() =
[time.[0] .. 0.1 .. 8.] |> List.map (fun x -> x, gradientFunctionMmf x) |> Chart.Line
let inflectionPointXValue =
3.45
let maximalSlope =
gradientFunctionMmf inflectionPointXValue
log(2.) / maximalSlope
let generationMmf =
sprintf "The generation time (MMF) is: %.1f min" (generationtimeMmf (vector [|16.46099291; 24.00147463; 0.2500698772; 3.741048641|]) * 60.)
let fittedValuesMMF =
// The parameter were determined locally for saving time during build processes
let f = Table.GrowthModels.morganMercerFlodin.GetFunctionValue (vector [|16.46099291; 24.00147463; 0.2500698772; 3.741048641|])
[time.[0] .. 0.1 .. Seq.last time]
|> Seq.map (fun x ->
x,f x
)
|> Chart.Line
let fittedChartMMF =
[
Chart.Point(time,cellCountLn)
|> Chart.withTraceInfo "log count"
fittedValuesMMF |> Chart.withTraceInfo "morganMercerFlodin"
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
|> Chart.withTitle "morganMercerFlodin"
"The generation time (MMF) is: 21.9 min"
|
Since this model expects a x axis crossing of the data it cannot be applied to the given data.
Parameters:
- l: upper asymptote
- k: growth rate
- t0: x axis crossing
let combinedGrowthChart =
[
Chart.Point(time,cellCountLn) |> Chart.withTraceInfo "log count"
Chart.Line(fittedValues)|> Chart.withTraceInfo "regression line"
fittedValuesGompertz |> Chart.withTraceInfo "Gompertz"
fittedValuesRichards |> Chart.withTraceInfo "Richards"
fittedValuesWeibull |> Chart.withTraceInfo "Weibull"
fittedValuesJanoschek |> Chart.withTraceInfo "Janoschek"
fittedValuesExp |> Chart.withTraceInfo "Exponential"
fittedValuesVerhulst |> Chart.withTraceInfo "Verhulst"
fittedValuesMMF |> Chart.withTraceInfo "MorganMercerFlodin"
]
|> Chart.combine
|> Chart.withTemplate ChartTemplates.lightMirrored
|> Chart.withXAxisStyle "time (h)"
|> Chart.withYAxisStyle "ln(cells/ml)"
let generationTimeTable =
let header = ["<b>Model</b>";"<b>Generation time (min)"]
let rows =
[
["manual selection (regression line)"; sprintf "%.1f" ((log(2.)/1.5150)* 60.)]
["Gompertz"; sprintf "%.1f" (60. * (generationtime gompertzParams log))]
["Richards"; sprintf "%.1f" (generationtimeRichards (vector [|23.25211263; 7.053516315; 5.646889803; 111.0132522|]) * 60.)]
["Weibull"; sprintf "%.1f" (generationtimeWeibull (vector [|16.40632433; 23.35537293; 0.2277752116; 2.900806071|]) * 60.) ]
["Janoschek"; sprintf "%.1f" (generationtimeJanoschek (vector [|16.40633962; 23.35535182; 0.01368422994; 2.900857027|]) * 60.)]
["Exponential"; sprintf "%.1f" (generationtimeExponential (vector [|4.813988967; 24.39950361; 0.3939132175|]) * 60.)]
["Verhulst"; sprintf "%.1f" (generationtimeVerhulst (vector [|23.39504328; 3.577488116; 1.072136278; 15.77380824|]) * 60.)]
["MMF"; sprintf "%.1f" (generationtimeMmf (vector [|16.46099291; 24.00147463; 0.2500698772; 3.741048641|]) * 60.)]
]
Chart.Table(
header,
rows,
HeaderFillColor = Color.fromHex "#45546a",
CellsFillColor = Color.fromColors [Color.fromHex "#deebf7";Color.fromString "lightgrey"]
)
namespace Plotly
namespace Plotly.NET
module Defaults
from Plotly.NET
<summary>
Contains mutable global default values.
Changing these values will apply the default values to all consecutive Chart generations.
</summary>
val mutable DefaultDisplayOptions: DisplayOptions
Multiple items
type DisplayOptions =
inherit DynamicObj
new: unit -> DisplayOptions
static member addAdditionalHeadTags: additionalHeadTags: XmlNode list -> (DisplayOptions -> DisplayOptions)
static member addDescription: description: XmlNode list -> (DisplayOptions -> DisplayOptions)
static member combine: first: DisplayOptions -> second: DisplayOptions -> DisplayOptions
static member getAdditionalHeadTags: displayOpts: DisplayOptions -> XmlNode list
static member getDescription: displayOpts: DisplayOptions -> XmlNode list
static member getPlotlyReference: displayOpts: DisplayOptions -> PlotlyJSReference
static member init: ?AdditionalHeadTags: XmlNode list * ?Description: XmlNode list * ?PlotlyJSReference: PlotlyJSReference -> DisplayOptions
static member initCDNOnly: unit -> DisplayOptions
...
--------------------
new: unit -> DisplayOptions
static member DisplayOptions.init: ?AdditionalHeadTags: Giraffe.ViewEngine.HtmlElements.XmlNode list * ?Description: Giraffe.ViewEngine.HtmlElements.XmlNode list * ?PlotlyJSReference: PlotlyJSReference -> DisplayOptions
type PlotlyJSReference =
| CDN of string
| Full
| Require of string
| NoReference
<summary>
Sets how plotly is referenced in the head of html docs.
</summary>
union case PlotlyJSReference.NoReference: PlotlyJSReference
namespace System
Multiple items
namespace FSharp
--------------------
namespace Microsoft.FSharp
namespace FSharp.Stats
namespace FSharp.Stats.Fitting
module NonLinearRegression
from FSharp.Stats.Fitting
Multiple items
module LinearRegression
from FSharp.Stats.Fitting
<summary>
Linear regression is used to estimate the relationship of one variable (y) with another (x) by expressing y in terms of a linear function of x.
</summary>
--------------------
type LinearRegression =
new: unit -> LinearRegression
static member fit: xData: vector * yData: Vector<float> * ?FittingMethod: Method * ?Constraint: Constraint<float * float> * ?Weighting: vector -> Coefficients + 1 overload
static member predict: coeff: Coefficients -> xValue: float -> float
static member predictMultivariate: coeff: Coefficients -> xVector: vector -> float
<summary>
This LinearRegression type summarized the most common fitting procedures.
</summary>
<returns>Either linear regression coefficients or a prediction function to map x values/vectors to its corresponding y value.</returns>
<example><code>
// e.g. days since experiment start
let xData = vector [|1. .. 100.|]
// e.g. plant size in cm
let yData = vector [|4.;7.;8.;9.;7.;11.; ...|]
// Estimate the intercept and slope of a line, that fits the data.
let coefficientsSimpleLinear =
LinearRegression.fit(xData,yData,FittingMethod=Fitting.Method.SimpleLinear,Constraint=Fitting.Constraint.RegressionThroughOrigin)
// Predict the size on day 10.5
LinearRegression.predict(coefficientsSimpleLinear) 10.5
</code></example>
--------------------
new: unit -> Fitting.LinearRegression
val time: float[]
val cellCount: float[]
val cellCountLn: float[]
Multiple items
type Array =
new: unit -> Array
static member geomspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float array
static member linspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float[]
--------------------
new: unit -> Array
val map: mapping: ('T -> 'U) -> array: 'T[] -> 'U[]
<summary>Builds a new array whose elements are the results of applying the given function
to each of the elements of the array.</summary>
<param name="mapping">The function to transform elements of the array.</param>
<param name="array">The input array.</param>
<returns>The array of transformed elements.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input array is null.</exception>
<example id="map-1"><code lang="fsharp">
let inputs = [| "a"; "bbb"; "cc" |]
inputs |> Array.map (fun x -> x.Length)
</code>
Evaluates to <c>[| 1; 3; 2 |]</c></example>
val log: value: 'T -> 'T (requires member Log)
<summary>Natural logarithm of the given number</summary>
<param name="value">The input value.</param>
<returns>The natural logarithm of the input.</returns>
<example id="log-example"><code lang="fsharp">
let logBase baseNumber value = (log value) / (log baseNumber)
logBase 2.0 32.0 // Evaluates to 5.0
logBase 10.0 1000.0 // Evaluates to 3.0
</code></example>
val chartOrig: GenericChart.GenericChart
type Chart =
static member AnnotatedHeatmap: zData: seq<#seq<'a1>> * annotationText: seq<#seq<string>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?X: seq<'a3> * ?MultiX: seq<seq<'a3>> * ?XGap: int * ?Y: seq<'a4> * ?MultiY: seq<seq<'a4>> * ?YGap: int * ?Text: 'a5 * ?MultiText: seq<'a5> * ?ColorBar: ColorBar * ?ColorScale: Colorscale * ?ShowScale: bool * ?ReverseScale: bool * ?ZSmooth: SmoothAlg * ?Transpose: bool * ?UseWebGL: bool * ?ReverseYAxis: bool * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a3 :> IConvertible and 'a4 :> IConvertible and 'a5 :> IConvertible) + 1 overload
static member Area: x: seq<#IConvertible> * y: seq<#IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: MarkerSymbol * ?MultiMarkerSymbol: seq<MarkerSymbol> * ?Marker: Marker * ?LineColor: Color * ?LineColorScale: Colorscale * ?LineWidth: float * ?LineDash: DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: Orientation * ?GroupNorm: GroupNorm * ?FillColor: Color * ?FillPatternShape: PatternShape * ?FillPattern: Pattern * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart (requires 'a2 :> IConvertible) + 1 overload
static member Bar: values: seq<#IConvertible> * ?Keys: seq<'a1> * ?MultiKeys: seq<seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerPatternShape: PatternShape * ?MultiMarkerPatternShape: seq<PatternShape> * ?MarkerPattern: Pattern * ?Marker: Marker * ?Base: #IConvertible * ?Width: 'a4 * ?MultiWidth: seq<'a4> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a4 :> IConvertible) + 1 overload
static member BoxPlot: ?X: seq<'a0> * ?MultiX: seq<seq<'a0>> * ?Y: seq<'a1> * ?MultiY: seq<seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Text: 'a2 * ?MultiText: seq<'a2> * ?FillColor: Color * ?MarkerColor: Color * ?Marker: Marker * ?Opacity: float * ?WhiskerWidth: float * ?BoxPoints: BoxPoints * ?BoxMean: BoxMean * ?Jitter: float * ?PointPos: float * ?Orientation: Orientation * ?OutlineColor: Color * ?OutlineWidth: float * ?Outline: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?Notched: bool * ?NotchWidth: float * ?QuartileMethod: QuartileMethod * ?UseDefaults: bool -> GenericChart (requires 'a0 :> IConvertible and 'a1 :> IConvertible and 'a2 :> IConvertible) + 2 overloads
static member Bubble: x: seq<#IConvertible> * y: seq<#IConvertible> * sizes: seq<int> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: MarkerSymbol * ?MultiMarkerSymbol: seq<MarkerSymbol> * ?Marker: Marker * ?LineColor: Color * ?LineColorScale: Colorscale * ?LineWidth: float * ?LineDash: DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: Orientation * ?GroupNorm: GroupNorm * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart (requires 'a2 :> IConvertible) + 1 overload
static member Candlestick: ``open`` : seq<#IConvertible> * high: seq<#IConvertible> * low: seq<#IConvertible> * close: seq<#IConvertible> * ?X: seq<'a4> * ?MultiX: seq<seq<'a4>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?Text: 'a5 * ?MultiText: seq<'a5> * ?Line: Line * ?IncreasingColor: Color * ?Increasing: FinanceMarker * ?DecreasingColor: Color * ?Decreasing: FinanceMarker * ?WhiskerWidth: float * ?ShowXAxisRangeSlider: bool * ?UseDefaults: bool -> GenericChart (requires 'a4 :> IConvertible and 'a5 :> IConvertible) + 2 overloads
static member Column: values: seq<#IConvertible> * ?Keys: seq<'a1> * ?MultiKeys: seq<seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?MarkerColor: Color * ?MarkerColorScale: Colorscale * ?MarkerOutline: Line * ?MarkerPatternShape: PatternShape * ?MultiMarkerPatternShape: seq<PatternShape> * ?MarkerPattern: Pattern * ?Marker: Marker * ?Base: #IConvertible * ?Width: 'a4 * ?MultiWidth: seq<'a4> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a4 :> IConvertible) + 1 overload
static member Contour: zData: seq<#seq<'a1>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?X: seq<'a2> * ?MultiX: seq<seq<'a2>> * ?Y: seq<'a3> * ?MultiY: seq<seq<'a3>> * ?Text: 'a4 * ?MultiText: seq<'a4> * ?ColorBar: ColorBar * ?ColorScale: Colorscale * ?ShowScale: bool * ?ReverseScale: bool * ?Transpose: bool * ?ContourLineColor: Color * ?ContourLineDash: DrawingStyle * ?ContourLineSmoothing: float * ?ContourLine: Line * ?ContoursColoring: ContourColoring * ?ContoursOperation: ConstraintOperation * ?ContoursType: ContourType * ?ShowContourLabels: bool * ?ContourLabelFont: Font * ?Contours: Contours * ?FillColor: Color * ?NContours: int * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a3 :> IConvertible and 'a4 :> IConvertible)
static member Funnel: x: seq<#IConvertible> * y: seq<#IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?Width: float * ?Offset: float * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: TextPosition * ?MultiTextPosition: seq<TextPosition> * ?Orientation: Orientation * ?AlignmentGroup: string * ?OffsetGroup: string * ?MarkerColor: Color * ?MarkerOutline: Line * ?Marker: Marker * ?TextInfo: TextInfo * ?ConnectorLineColor: Color * ?ConnectorLineStyle: DrawingStyle * ?ConnectorFillColor: Color * ?ConnectorLine: Line * ?Connector: FunnelConnector * ?InsideTextFont: Font * ?OutsideTextFont: Font * ?UseDefaults: bool -> GenericChart (requires 'a2 :> IConvertible)
static member Heatmap: zData: seq<#seq<'a1>> * ?X: seq<'a2> * ?MultiX: seq<seq<'a2>> * ?Y: seq<'a3> * ?MultiY: seq<seq<'a3>> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?XGap: int * ?YGap: int * ?Text: 'a4 * ?MultiText: seq<'a4> * ?ColorBar: ColorBar * ?ColorScale: Colorscale * ?ShowScale: bool * ?ReverseScale: bool * ?ZSmooth: SmoothAlg * ?Transpose: bool * ?UseWebGL: bool * ?ReverseYAxis: bool * ?UseDefaults: bool -> GenericChart (requires 'a1 :> IConvertible and 'a2 :> IConvertible and 'a3 :> IConvertible and 'a4 :> IConvertible) + 1 overload
...
static member Chart.Point: xy: seq<#IConvertible * #IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'a2 :> IConvertible)
static member Chart.Point: x: seq<#IConvertible> * y: seq<#IConvertible> * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'c * ?MultiText: seq<'c> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'c :> IConvertible)
static member Chart.withTraceInfo: ?Name: string * ?Visible: StyleParam.Visible * ?ShowLegend: bool * ?LegendRank: int * ?LegendGroup: string * ?LegendGroupTitle: Title -> (GenericChart.GenericChart -> GenericChart.GenericChart)
static member Chart.withTemplate: template: Template -> (GenericChart.GenericChart -> GenericChart.GenericChart)
module ChartTemplates
from Plotly.NET
val lightMirrored: Template
static member Chart.withYAxisStyle: ?TitleText: string * ?TitleFont: Font * ?TitleStandoff: int * ?Title: Title * ?Color: Color * ?AxisType: StyleParam.AxisType * ?MinMax: (#IConvertible * #IConvertible) * ?Mirror: StyleParam.Mirror * ?ShowSpikes: bool * ?SpikeColor: Color * ?SpikeThickness: int * ?ShowLine: bool * ?LineColor: Color * ?ShowGrid: bool * ?GridColor: Color * ?GridDash: StyleParam.DrawingStyle * ?ZeroLine: bool * ?ZeroLineColor: Color * ?Anchor: StyleParam.LinearAxisId * ?Side: StyleParam.Side * ?Overlaying: StyleParam.LinearAxisId * ?AutoShift: bool * ?Shift: int * ?Domain: (float * float) * ?Position: float * ?CategoryOrder: StyleParam.CategoryOrder * ?CategoryArray: seq<#IConvertible> * ?RangeSlider: LayoutObjects.RangeSlider * ?RangeSelector: LayoutObjects.RangeSelector * ?BackgroundColor: Color * ?ShowBackground: bool * ?Id: StyleParam.SubPlotId -> (GenericChart.GenericChart -> GenericChart.GenericChart)
val chartLog: GenericChart.GenericChart
static member Chart.withXAxisStyle: ?TitleText: string * ?TitleFont: Font * ?TitleStandoff: int * ?Title: Title * ?Color: Color * ?AxisType: StyleParam.AxisType * ?MinMax: (#IConvertible * #IConvertible) * ?Mirror: StyleParam.Mirror * ?ShowSpikes: bool * ?SpikeColor: Color * ?SpikeThickness: int * ?ShowLine: bool * ?LineColor: Color * ?ShowGrid: bool * ?GridColor: Color * ?GridDash: StyleParam.DrawingStyle * ?ZeroLine: bool * ?ZeroLineColor: Color * ?Anchor: StyleParam.LinearAxisId * ?Side: StyleParam.Side * ?Overlaying: StyleParam.LinearAxisId * ?Domain: (float * float) * ?Position: float * ?CategoryOrder: StyleParam.CategoryOrder * ?CategoryArray: seq<#IConvertible> * ?RangeSlider: LayoutObjects.RangeSlider * ?RangeSelector: LayoutObjects.RangeSelector * ?BackgroundColor: Color * ?ShowBackground: bool * ?Id: StyleParam.SubPlotId -> (GenericChart.GenericChart -> GenericChart.GenericChart)
val growthChart: GenericChart.GenericChart
static member Chart.Grid: ?SubPlots: (StyleParam.LinearAxisId * StyleParam.LinearAxisId)[][] * ?XAxes: StyleParam.LinearAxisId[] * ?YAxes: StyleParam.LinearAxisId[] * ?RowOrder: StyleParam.LayoutGridRowOrder * ?Pattern: StyleParam.LayoutGridPattern * ?XGap: float * ?YGap: float * ?Domain: LayoutObjects.Domain * ?XSide: StyleParam.LayoutGridXSide * ?YSide: StyleParam.LayoutGridYSide -> (#seq<'a1> -> GenericChart.GenericChart) (requires 'a1 :> seq<GenericChart.GenericChart>)
static member Chart.Grid: nRows: int * nCols: int * ?SubPlots: (StyleParam.LinearAxisId * StyleParam.LinearAxisId)[][] * ?XAxes: StyleParam.LinearAxisId[] * ?YAxes: StyleParam.LinearAxisId[] * ?RowOrder: StyleParam.LayoutGridRowOrder * ?Pattern: StyleParam.LayoutGridPattern * ?XGap: float * ?YGap: float * ?Domain: LayoutObjects.Domain * ?XSide: StyleParam.LayoutGridXSide * ?YSide: StyleParam.LayoutGridYSide -> (#seq<GenericChart.GenericChart> -> GenericChart.GenericChart)
module GenericChart
from Plotly.NET
<summary>
Module to represent a GenericChart
</summary>
val toChartHTML: gChart: GenericChart.GenericChart -> string
val logPhaseX: Vector<float>
Multiple items
val vector: l: seq<float> -> Vector<float>
--------------------
type vector = Vector<float>
val logPhaseY: Vector<float>
val regressionCoeffs: Coefficients
module OLS
from FSharp.Stats.Fitting.LinearRegressionModule
<summary>
Ordinary Least Squares (OLS) regression aims to minimise the sum of squared y intercepts between the original and predicted points at each x value.
</summary>
module Linear
from FSharp.Stats.Fitting.LinearRegressionModule.OLS
<summary>
Simple linear regression using straight lines: f(x) = a + bx.
</summary>
module Univariable
from FSharp.Stats.Fitting.LinearRegressionModule.OLS.Linear
<summary>
Univariable handles two dimensional x,y data.
</summary>
val fit: xData: Vector<float> -> yData: Vector<float> -> Coefficients
<summary>
Calculates the intercept and slope for a straight line fitting the data. Linear regression minimizes the sum of squared residuals.
</summary>
<param name="xData">vector of x values</param>
<param name="yData">vector of y values</param>
<returns>vector of [intercept; slope]</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|1.;2.;3.;4.;5.;6.|]
// e.g. some measured feature
let yData = vector [|4.;7.;9.;10.;11.;15.|]
// Estimate the coefficients of a straight line fitting the given data
let coefficients =
Univariable.fit xData yData
</code></example>
val slope: float
val generationTime: float
val fittedValues: seq<float * float>
val f: (float -> float)
val predict: coef: Coefficients -> x: float -> float
<summary>
Takes intercept and slope of simple linear regression to predict the corresponding y value.
</summary>
<param name="coef">vector of [intercept;slope] (e.g. determined by Univariable.coefficient)</param>
<param name="x">x value of which the corresponding y value should be predicted</param>
<returns>predicted y value with given coefficients at X=x</returns>
<example><code>
// e.g. days since a certain event
let xData = vector [|1.;2.;3.;4.;5.;6.|]
// e.g. some measured feature
let yData = vector [|4.;7.;9.;10.;11.;15.|]
// Estimate the coefficients of a straight line fitting the given data
let coefficients =
Univariable.fit xData yData
// Predict the feature at midnight between day 1 and 2.
Univariable.predict coefficients 1.5
</code></example>
Multiple items
type Coefficients =
new: coefficients: vector -> Coefficients
member Item: degree: int -> float
member Predict: x: float -> float + 1 overload
override ToString: unit -> string
member getCoefficient: degree: int -> float
static member Empty: unit -> Coefficients
static member Init: coefficients: vector -> Coefficients
member Coefficients: vector
member Constant: float
member Count: int
...
<summary>
Polynomial coefficients with various properties are stored within this type.
</summary>
--------------------
new: coefficients: vector -> Coefficients
Multiple items
module Seq
from FSharp.Stats
<summary>
Module to compute common statistical measure
</summary>
--------------------
module Seq
from Microsoft.FSharp.Collections
<summary>Contains operations for working with values of type <see cref="T:Microsoft.FSharp.Collections.seq`1" />.</summary>
--------------------
type Seq =
new: unit -> Seq
static member geomspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> seq<float>
static member linspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> seq<float>
--------------------
new: unit -> Seq
val map: mapping: ('T -> 'U) -> source: seq<'T> -> seq<'U>
<summary>Builds a new collection whose elements are the results of applying the given function
to each of the elements of the collection. The given function will be applied
as elements are demanded using the <c>MoveNext</c> method on enumerators retrieved from the
object.</summary>
<remarks>The returned sequence may be passed between threads safely. However,
individual IEnumerator values generated from the returned sequence should not be accessed concurrently.</remarks>
<param name="mapping">A function to transform items from the input sequence.</param>
<param name="source">The input sequence.</param>
<returns>The result sequence.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input sequence is null.</exception>
<example id="item-1"><code lang="fsharp">
let inputs = ["a"; "bbb"; "cc"]
inputs |> Seq.map (fun x -> x.Length)
</code>
Evaluates to a sequence yielding the same results as <c>seq { 1; 3; 2 }</c></example>
val x: float
val chartLinearRegression: GenericChart.GenericChart
static member Chart.Line: xy: seq<#IConvertible * #IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'c * ?MultiText: seq<'c> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?LineColor: Color * ?LineColorScale: StyleParam.Colorscale * ?LineWidth: float * ?LineDash: StyleParam.DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?Fill: StyleParam.Fill * ?FillColor: Color * ?FillPattern: TraceObjects.Pattern * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'c :> IConvertible)
static member Chart.Line: x: seq<#IConvertible> * y: seq<#IConvertible> * ?ShowMarkers: bool * ?Name: string * ?ShowLegend: bool * ?Opacity: float * ?MultiOpacity: seq<float> * ?Text: 'a2 * ?MultiText: seq<'a2> * ?TextPosition: StyleParam.TextPosition * ?MultiTextPosition: seq<StyleParam.TextPosition> * ?MarkerColor: Color * ?MarkerColorScale: StyleParam.Colorscale * ?MarkerOutline: Line * ?MarkerSymbol: StyleParam.MarkerSymbol * ?MultiMarkerSymbol: seq<StyleParam.MarkerSymbol> * ?Marker: TraceObjects.Marker * ?LineColor: Color * ?LineColorScale: StyleParam.Colorscale * ?LineWidth: float * ?LineDash: StyleParam.DrawingStyle * ?Line: Line * ?AlignmentGroup: string * ?OffsetGroup: string * ?StackGroup: string * ?Orientation: StyleParam.Orientation * ?GroupNorm: StyleParam.GroupNorm * ?Fill: StyleParam.Fill * ?FillColor: Color * ?FillPattern: TraceObjects.Pattern * ?UseWebGL: bool * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'a2 :> IConvertible)
static member Chart.combine: gCharts: seq<GenericChart.GenericChart> -> GenericChart.GenericChart
val generationTimeManual: string
val sprintf: format: Printf.StringFormat<'T> -> 'T
<summary>Print to a string using the given format.</summary>
<param name="format">The formatter.</param>
<returns>The formatted result.</returns>
<example>See <c>Printf.sprintf</c> (link: <see cref="M:Microsoft.FSharp.Core.PrintfModule.PrintFormatToStringThen``1" />) for examples.</example>
val solverOptions: xData: float[] -> yDataLog: float[] -> expectedGenerationTime: float -> usedLogTransform: (float -> float) -> SolverOptions
val xData: float[]
Multiple items
val float: value: 'T -> float (requires member op_Explicit)
<summary>Converts the argument to 64-bit float. This is a direct conversion for all
primitive numeric types. For strings, the input is converted using <c>Double.Parse()</c>
with InvariantCulture settings. Otherwise the operation requires an appropriate
static conversion method on the input type.</summary>
<param name="value">The input value.</param>
<returns>The converted float</returns>
<example id="float-example"><code lang="fsharp"></code></example>
--------------------
[<Struct>]
type float = Double
<summary>An abbreviation for the CLI type <see cref="T:System.Double" />.</summary>
<category>Basic Types</category>
--------------------
type float<'Measure> =
float
<summary>The type of double-precision floating point numbers, annotated with a unit of measure.
The unit of measure is erased in compiled code and when values of this type
are analyzed using reflection. The type is representationally equivalent to
<see cref="T:System.Double" />.</summary>
<category index="6">Basic Types with Units of Measure</category>
val yDataLog: float[]
val expectedGenerationTime: float
val usedLogTransform: (float -> float)
val a: float
val min: source: seq<'T> -> 'T (requires comparison)
<summary>Returns the lowest of all elements of the sequence, compared via <c>Operators.min</c>.</summary>
<param name="source">The input sequence.</param>
<returns>The smallest element of the sequence.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input sequence is null.</exception>
<exception cref="T:System.ArgumentException">Thrown when the input sequence is empty.</exception>
<example id="min-1"><code lang="fsharp">
let inputs = [10; 12; 11]
inputs |> Seq.min
</code>
Evaluates to <c>10</c></example>
<example id="min-2"><code lang="fsharp">
let inputs = []
inputs |> Seq.min
</code>
Throws <c>System.ArgumentException</c>.
</example>
val c: float
val max: source: seq<'T> -> 'T (requires comparison)
<summary>Returns the greatest of all elements of the sequence, compared via Operators.max</summary>
<param name="source">The input sequence.</param>
<exception cref="T:System.ArgumentNullException">Thrown when the input sequence is null.</exception>
<exception cref="T:System.ArgumentException">Thrown when the input sequence is empty.</exception>
<returns>The largest element of the sequence.</returns>
<example id="max-1"><code lang="fsharp">
let inputs = [ 10; 12; 11 ]
inputs |> Seq.max
</code>
Evaluates to <c>12</c></example>
<example id="max-2"><code lang="fsharp">
let inputs = [ ]
inputs |> Seq.max
</code>
Throws <c>System.ArgumentException</c>.
</example>
val b: float
type Math =
static member Abs: value: decimal -> decimal + 7 overloads
static member Acos: d: float -> float
static member Acosh: d: float -> float
static member Asin: d: float -> float
static member Asinh: d: float -> float
static member Atan: d: float -> float
static member Atan2: y: float * x: float -> float
static member Atanh: d: float -> float
static member BigMul: a: int * b: int -> int64 + 2 overloads
static member BitDecrement: x: float -> float
...
<summary>Provides constants and static methods for trigonometric, logarithmic, and other common mathematical functions.</summary>
field Math.E: float = 2.71828182846
<summary>Represents the natural logarithmic base, specified by the constant, <see langword="e" />.</summary>
val m: float
val yAtInflection: float
val zip: source1: seq<'T1> -> source2: seq<'T2> -> seq<'T1 * 'T2>
<summary>Combines the two sequences into a sequence of pairs. The two sequences need not have equal lengths:
when one sequence is exhausted any remaining elements in the other
sequence are ignored.</summary>
<param name="source1">The first input sequence.</param>
<param name="source2">The second input sequence.</param>
<returns>The result sequence.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when either of the input sequences is null.</exception>
<example id="zip-1"><code lang="fsharp">
let numbers = [1; 2]
let names = ["one"; "two"]
Seq.zip numbers names
</code>
Evaluates to a sequence yielding the same results as <c>seq { (1, "one"); (2, "two") }</c>.
</example>
val minBy: projection: ('T -> 'U) -> source: seq<'T> -> 'T (requires comparison)
<summary>Returns the lowest of all elements of the sequence, compared via Operators.min on the function result.</summary>
<param name="projection">A function to transform items from the input sequence into comparable keys.</param>
<param name="source">The input sequence.</param>
<returns>The smallest element of the sequence.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input sequence is null.</exception>
<exception cref="T:System.ArgumentException">Thrown when the input sequence is empty.</exception>
<example id="minby-1"><code lang="fsharp">
let inputs = [ "aaa"; "b"; "cccc" ]
inputs |> Seq.minBy (fun s -> s.Length)
</code>
Evaluates to <c>"b"</c></example>
<example id="minby-2"><code lang="fsharp">
let inputs = []
inputs |> Seq.minBy (fun (s: string) -> s.Length)
</code>
Throws <c>System.ArgumentException</c>.
</example>
val xValue: float
val yValue: float
Math.Abs(value: float32) : float32
Math.Abs(value: sbyte) : sbyte
Math.Abs(value: nativeint) : nativeint
Math.Abs(value: int64) : int64
Math.Abs(value: int) : int
Math.Abs(value: int16) : int16
Math.Abs(value: float) : float
Math.Abs(value: decimal) : decimal
val fst: tuple: ('T1 * 'T2) -> 'T1
<summary>Return the first element of a tuple, <c>fst (a,b) = a</c>.</summary>
<param name="tuple">The input tuple.</param>
<returns>The first value.</returns>
<example id="fst-example"><code lang="fsharp">
fst ("first", 2) // Evaluates to "first"
</code></example>
val createSolverOption: minimumDeltaValue: float -> minimumDeltaParameters: float -> maximumIterations: int -> initialParamGuess: float[] -> SolverOptions
val gompertzParams: vector
module LevenbergMarquardt
from FSharp.Stats.Fitting.NonLinearRegression
val estimatedParams: model: Model -> solverOptions: SolverOptions -> lambdaInitial: float -> lambdaFactor: float -> xData: float[] -> yData: float[] -> vector
<summary>Returns a parameter vector as a possible solution for least square based nonlinear fitting of a given dataset (xData, yData) with a given <br />model function. </summary>
<remarks></remarks>
<param name="model"></param>
<param name="solverOptions"></param>
<param name="lambdaInitial"></param>
<param name="lambdaFactor"></param>
<param name="xData"></param>
<param name="yData"></param>
<returns></returns>
<example><code></code></example>
module Table
from FSharp.Stats.Fitting.NonLinearRegression
module GrowthModels
from FSharp.Stats.Fitting.NonLinearRegression.Table
val gompertz: Model
<summary>
The gompertz function describes the log cell count at time point t.
</summary>
val fittingFunction: (float -> float)
val fittedValuesGompertz: GenericChart.GenericChart
val last: source: seq<'T> -> 'T
<summary>Returns the last element of the sequence.</summary>
<param name="source">The input sequence.</param>
<returns>The last element of the sequence.</returns>
<exception cref="T:System.ArgumentNullException">Thrown when the input sequence is null.</exception>
<exception cref="T:System.ArgumentException">Thrown when the input does not have any elements.</exception>
<example id="last-1"><code lang="fsharp">
["pear"; "banana"] |> Seq.last
</code>
Evaluates to <c>banana</c></example>
<example id="last-2"><code lang="fsharp">
[] |> Seq.last
</code>
Throws <c>ArgumentException</c></example>
val fittedChartGompertz: GenericChart.GenericChart
val generationtime: parametervector: vector -> logTransform: (float -> float) -> float
val parametervector: vector
val logTransform: (float -> float)
val lag: parametervector: vector -> float
val g: string
val l: string
val richardsParams: vector
val richards: Model
<summary>
4 parameter richards curve with minimum at 0; d &lt;&gt; 1
</summary>
val fittingFunctionRichards: (float -> float)
val generationtimeRichards: richardParameters: vector -> float
val richardParameters: vector
val l: float
val k: float
val y: float
val d: float
val gradientFunctionRichards: (float -> float)
val t: float
Math.Exp(d: float) : float
val maximalSlope: float
val fittedValuesRichards: GenericChart.GenericChart
val fittedChartRichards: GenericChart.GenericChart
val fittedChartRichardsS: GenericChart.GenericChart
static member Chart.withTitle: title: Title -> (GenericChart.GenericChart -> GenericChart.GenericChart)
static member Chart.withTitle: title: string * ?TitleFont: Font -> (GenericChart.GenericChart -> GenericChart.GenericChart)
val generationRichards: string
val weibullParams: vector
val weibull: Model
<summary>
weibull growth model; if d=1 then it is a simple exponential growth model
</summary>
val fittingFunctionWeibull: (float -> float)
val generationtimeWeibull: weibullParameters: vector -> float
val weibullParameters: vector
val gradientFunctionWeibull: (float -> float)
val inflectionPointXValue: float
val fittedValuesWeibull: GenericChart.GenericChart
val fittedChartWeibull: GenericChart.GenericChart
val fittedChartWeibullS: GenericChart.GenericChart
val generationWeibull: string
val janoschekParams: vector
val janoschek: Model
<summary>
stable growth model similar to weibull model
</summary>
val fittingFunctionJanoschek: (float -> float)
val generationtimeJanoschek: janoschekParameters: vector -> float
val janoschekParameters: vector
val gradientFunctionJanoschek: (float -> float)
val slopeChart: (unit -> GenericChart.GenericChart)
Multiple items
module List
from FSharp.Stats
<summary>
Module to compute common statistical measure on list
</summary>
--------------------
module List
from Microsoft.FSharp.Collections
<summary>Contains operations for working with values of type <see cref="T:Microsoft.FSharp.Collections.list`1" />.</summary>
<namespacedoc><summary>Operations for collections such as lists, arrays, sets, maps and sequences. See also
<a href="https://docs.microsoft.com/dotnet/fsharp/language-reference/fsharp-collection-types">F# Collection Types</a> in the F# Language Guide.
</summary></namespacedoc>
--------------------
type List =
new: unit -> List
static member geomspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float list
static member linspace: start: float * stop: float * num: int * ?IncludeEndpoint: bool -> float list
--------------------
type List<'T> =
| op_Nil
| op_ColonColon of Head: 'T * Tail: 'T list
interface IReadOnlyList<'T>
interface IReadOnlyCollection<'T>
interface IEnumerable
interface IEnumerable<'T>
member GetReverseIndex: rank: int * offset: int -> int
member GetSlice: startIndex: int option * endIndex: int option -> 'T list
static member Cons: head: 'T * tail: 'T list -> 'T list
member Head: 'T
member IsEmpty: bool
member Item: index: int -> 'T with get
...
<summary>The type of immutable singly-linked lists.</summary>
<remarks>Use the constructors <c>[]</c> and <c>::</c> (infix) to create values of this type, or
the notation <c>[1;2;3]</c>. Use the values in the <c>List</c> module to manipulate
values of this type, or pattern match against the values directly.
</remarks>
<exclude />
--------------------
new: unit -> List
val map: mapping: ('T -> 'U) -> list: 'T list -> 'U list
<summary>Builds a new collection whose elements are the results of applying the given function
to each of the elements of the collection.</summary>
<param name="mapping">The function to transform elements from the input list.</param>
<param name="list">The input list.</param>
<returns>The list of transformed elements.</returns>
<example id="map-1"><code lang="fsharp">
let inputs = [ "a"; "bbb"; "cc" ]
inputs |> List.map (fun x -> x.Length)
</code>
Evaluates to <c>[ 1; 3; 2 ]</c></example>
val fittedValuesJanoschek: GenericChart.GenericChart
val fittedChartJanoschek: GenericChart.GenericChart
val fittedChartJanoschekS: GenericChart.GenericChart
val generationJanoschek: string
val exponentialParams: vector
val exponential: Model
<summary>
exponential growth model
</summary>
val fittingFunctionExponential: (float -> float)
val generationtimeExponential: expParameters: vector -> float
val expParameters: vector
val gradientFunctionExponential: (float -> float)
val fittedValuesExp: GenericChart.GenericChart
val fittedChartExp: GenericChart.GenericChart
val fittedChartExpS: GenericChart.GenericChart
val generationExponential: string
val verhulstParams: vector
val verhulst4Param: Model
<summary>
4 parameter verhulst model with variably lowers asymptote
</summary>
val fittingFunctionVerhulst: unit -> (float -> float)
val verhulst: Model
<summary>
3 parameter verhulst logistic model with lower asymptote=0
</summary>
val generationtimeVerhulst: verhulstParameters: vector -> float
val verhulstParameters: vector
val lmax: float
val lmin: float
val gradientFunctionVerhulst: (float -> float)
val fittedValuesVerhulst: GenericChart.GenericChart
val fittedChartVerhulst: GenericChart.GenericChart
val fittedChartVerhulstS: GenericChart.GenericChart
val generationVerhulst: string
val morganMercerFlodinParams: vector
val morganMercerFlodin: Model
<summary>
4 parameter Morgan-Mercer-Flodin growth model
</summary>
val fittingFunctionMMF: unit -> (float -> float)
val generationtimeMmf: mmfParameters: vector -> float
val mmfParameters: vector
val gradientFunctionMmf: (float -> float)
val generationMmf: string
val fittedValuesMMF: GenericChart.GenericChart
val fittedChartMMF: GenericChart.GenericChart
val combinedGrowthChart: GenericChart.GenericChart
val generationTimeTable: GenericChart.GenericChart
val header: string list
val rows: string list list
static member Chart.Table: header: TraceObjects.TableHeader * cells: TraceObjects.TableCells * ?Name: string * ?ColumnOrder: seq<int> * ?ColumnWidth: float * ?MultiColumnWidth: seq<float> * ?UseDefaults: bool -> GenericChart.GenericChart
static member Chart.Table: headerValues: seq<#seq<'b>> * cellsValues: seq<#seq<'d>> * ?TransposeCells: bool * ?HeaderAlign: StyleParam.HorizontalAlign * ?HeaderMultiAlign: seq<StyleParam.HorizontalAlign> * ?HeaderFillColor: Color * ?HeaderHeight: int * ?HeaderOutlineColor: Color * ?HeaderOutlineWidth: float * ?HeaderOutlineMultiWidth: seq<float> * ?HeaderOutline: Line * ?CellsAlign: StyleParam.HorizontalAlign * ?CellsMultiAlign: seq<StyleParam.HorizontalAlign> * ?CellsFillColor: Color * ?CellsHeight: int * ?CellsOutlineColor: Color * ?CellsOutlineWidth: float * ?CellsOutlineMultiWidth: seq<float> * ?CellsOutline: Line * ?Name: string * ?ColumnOrder: seq<int> * ?ColumnWidth: float * ?MultiColumnWidth: seq<float> * ?UseDefaults: bool -> GenericChart.GenericChart (requires 'b :> IConvertible and 'd :> IConvertible)
type Color =
override Equals: other: obj -> bool
override GetHashCode: unit -> int
static member fromARGB: a: int -> r: int -> g: int -> b: int -> Color
static member fromColorScaleValues: c: seq<#IConvertible> -> Color
static member fromColors: c: seq<Color> -> Color
static member fromHex: s: string -> Color
static member fromKeyword: c: ColorKeyword -> Color
static member fromRGB: r: int -> g: int -> b: int -> Color
static member fromString: c: string -> Color
member Value: obj
<summary>
Plotly color can be a single color, a sequence of colors, or a sequence of numeric values referencing the color of the colorscale obj
</summary>
static member Color.fromHex: s: string -> Color
static member Color.fromColors: c: seq<Color> -> Color
static member Color.fromString: c: string -> Color
val explGompertz: model: Model -> coefs: Vector<float> -> GenericChart.GenericChart
val model: Model
type Model =
{
ParameterNames: string[]
GetFunctionValue: Vector<float> -> float -> float
GetGradientValue: Vector<float> -> Vector<float> -> float -> Vector<float>
}
val coefs: Vector<float>
val ff: (float -> float)
Model.GetFunctionValue: Vector<float> -> float -> float
<summary>
originally GetValue; contains function body
</summary>
val gom: GenericChart.GenericChart
val rich: GenericChart.GenericChart
val explRichGeneric: model: Model -> coefs: Vector<float> -> GenericChart.GenericChart
val richGeneric: GenericChart.GenericChart
val richardsGeneric: Model
<summary>
Generalized logistic function or curve, also known as Richards' curve with 7 parameters.
Logistic function of the form "Y(t) = A + (K - A) / (C + Q * e^(-B * (t - M)))**(1. / v)"
</summary>
val wei: GenericChart.GenericChart
val jan: GenericChart.GenericChart
val exp: GenericChart.GenericChart
val ver: GenericChart.GenericChart
val ver4: GenericChart.GenericChart
val mmf: GenericChart.GenericChart
val vonB: GenericChart.GenericChart
val vonBertalanffy: Model
<summary>
3 parameter von Bertalanffy growth model
</summary>